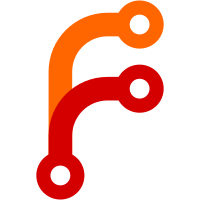
This is a git-remote-gcrypt encrypted special remote. Only sending files in to the remote works, and only for local repositories. Most of the work so far has involved making initremote work. A particular problem is that remote setup in this case needs to generate its own uuid, derivied from the gcrypt-id. That required some larger changes in the code to support. For ssh remotes, this will probably just reuse Remote.Rsync's code, so should be easy enough. And for downloading from a web remote, I will need to factor out the part of Remote.Git that does that. One particular thing that will need work is supporting hot-swapping a local gcrypt remote. I think it needs to store the gcrypt-id in the git config of the local remote, so that it can check it every time, and compare with the cached annex-uuid for the remote. If there is a mismatch, it can change both the cached annex-uuid and the gcrypt-id. That should work, and I laid some groundwork for it by already reading the remote's config when it's local. (Also needed for other reasons.) This commit was sponsored by Daniel Callahan.
56 lines
1.4 KiB
Haskell
56 lines
1.4 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2013 Joey Hess <joey@kitenet.net>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.EnableRemote where
|
|
|
|
import Common.Annex
|
|
import Command
|
|
import qualified Logs.Remote
|
|
import qualified Types.Remote as R
|
|
import qualified Command.InitRemote as InitRemote
|
|
|
|
import qualified Data.Map as M
|
|
|
|
def :: [Command]
|
|
def = [command "enableremote"
|
|
(paramPair paramName $ paramOptional $ paramRepeating paramKeyValue)
|
|
seek SectionSetup "enables use of an existing special remote"]
|
|
|
|
seek :: [CommandSeek]
|
|
seek = [withWords start]
|
|
|
|
start :: [String] -> CommandStart
|
|
start [] = unknownNameError "Specify the name of the special remote to enable."
|
|
start (name:ws) = go =<< InitRemote.findExisting name
|
|
where
|
|
config = Logs.Remote.keyValToConfig ws
|
|
|
|
go Nothing = unknownNameError "Unknown special remote name."
|
|
go (Just (u, c)) = do
|
|
let fullconfig = config `M.union` c
|
|
t <- InitRemote.findType fullconfig
|
|
|
|
showStart "enableremote" name
|
|
next $ perform t u fullconfig
|
|
|
|
unknownNameError :: String -> Annex a
|
|
unknownNameError prefix = do
|
|
names <- InitRemote.remoteNames
|
|
error $ prefix ++
|
|
if null names
|
|
then ""
|
|
else " Known special remotes: " ++ intercalate " " names
|
|
|
|
perform :: RemoteType -> UUID -> R.RemoteConfig -> CommandPerform
|
|
perform t u c = do
|
|
(c', u') <- R.setup t (Just u) c
|
|
next $ cleanup u' c'
|
|
|
|
cleanup :: UUID -> R.RemoteConfig -> CommandCleanup
|
|
cleanup u c = do
|
|
Logs.Remote.configSet u c
|
|
return True
|