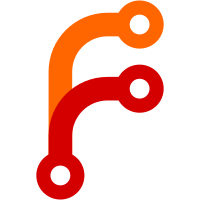
Started with a problem when running addurl on a really long url, because the whole url is munged into the filename. Ended up doing a fairly extensive review for places where filenames could get too large, although it's hard to say I'm not missed any.. Backend.Url had a 128 character limit, which is fine when the limit is 255, but not if it's a lot shorter on some systems. So check the pathconf() limit. Note that this could result in fromUrl creating different keys for the same url, if run on systems with different limits. I don't see this is likely to cause any problems. That can already happen when using addurl --fast, or if the content of an url changes. Both Command.AddUrl and Backend.Url assumed that urls don't contain a lot of multi-byte unicode, and would fail to truncate an url that did properly. A few places use a filename as the template to make a temp file. While that's nice in that the temp file name can be easily related back to the original filename, it could lead to `git annex add` failing to add a filename that was at or close to the maximum length. Note that in Command.Add.lockdown, the template is still derived from the filename, just with enough space left to turn it into a temp file. This is an important optimisation, because the assistant may lock down a bunch of files all at once, and using the same template for all of them would cause openTempFile to iterate through the same set of names, looking for an unused temp file. I'm not very happy with the relatedTemplate hack, but it avoids that slowdown. Backend.WORM does not limit the filename stored in the key. I have not tried to change that; so git annex add will fail on really long filenames when using the WORM backend. It seems better to preserve the invariant that a WORM key always contains the complete filename, since the filename is the only unique material in the key, other than mtime and size. Since nobody has complained about add failing (I think I saw it once?) on WORM, probably it's ok, or nobody but me uses it. There may be compatability problems if using git annex addurl --fast or the WORM backend on a system with the 255 limit and then trying to use that repo in a system with a smaller limit. I have not tried to deal with those. This commit was sponsored by Alexander Brem. Thanks!
174 lines
5.2 KiB
Haskell
174 lines
5.2 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2011-2013 Joey Hess <joey@kitenet.net>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.AddUrl where
|
|
|
|
import Network.URI
|
|
|
|
import Common.Annex
|
|
import Command
|
|
import Backend
|
|
import qualified Command.Add
|
|
import qualified Annex
|
|
import qualified Annex.Queue
|
|
import qualified Backend.URL
|
|
import qualified Utility.Url as Url
|
|
import Annex.Content
|
|
import Logs.Web
|
|
import qualified Option
|
|
import Types.Key
|
|
import Types.KeySource
|
|
import Config
|
|
import Annex.Content.Direct
|
|
import Logs.Location
|
|
import qualified Logs.Transfer as Transfer
|
|
import Utility.Daemon (checkDaemon)
|
|
|
|
def :: [Command]
|
|
def = [notBareRepo $ withOptions [fileOption, pathdepthOption, relaxedOption] $
|
|
command "addurl" (paramRepeating paramUrl) seek
|
|
SectionCommon "add urls to annex"]
|
|
|
|
fileOption :: Option
|
|
fileOption = Option.field [] "file" paramFile "specify what file the url is added to"
|
|
|
|
pathdepthOption :: Option
|
|
pathdepthOption = Option.field [] "pathdepth" paramNumber "path components to use in filename"
|
|
|
|
relaxedOption :: Option
|
|
relaxedOption = Option.flag [] "relaxed" "skip size check"
|
|
|
|
seek :: [CommandSeek]
|
|
seek = [withField fileOption return $ \f ->
|
|
withFlag relaxedOption $ \relaxed ->
|
|
withField pathdepthOption (return . maybe Nothing readish) $ \d ->
|
|
withStrings $ start relaxed f d]
|
|
|
|
start :: Bool -> Maybe FilePath -> Maybe Int -> String -> CommandStart
|
|
start relaxed optfile pathdepth s = go $ fromMaybe bad $ parseURI s
|
|
where
|
|
bad = fromMaybe (error $ "bad url " ++ s) $
|
|
parseURI $ escapeURIString isUnescapedInURI s
|
|
go url = do
|
|
pathmax <- liftIO $ fileNameLengthLimit "."
|
|
let file = fromMaybe (url2file url pathdepth pathmax) optfile
|
|
showStart "addurl" file
|
|
next $ perform relaxed s file
|
|
|
|
perform :: Bool -> String -> FilePath -> CommandPerform
|
|
perform relaxed url file = ifAnnexed file addurl geturl
|
|
where
|
|
geturl = next $ addUrlFile relaxed url file
|
|
addurl (key, _backend)
|
|
| relaxed = do
|
|
setUrlPresent key url
|
|
next $ return True
|
|
| otherwise = do
|
|
headers <- getHttpHeaders
|
|
ifM (liftIO $ Url.check url headers $ keySize key)
|
|
( do
|
|
setUrlPresent key url
|
|
next $ return True
|
|
, do
|
|
warning $ "failed to verify url: " ++ url
|
|
stop
|
|
)
|
|
|
|
addUrlFile :: Bool -> String -> FilePath -> Annex Bool
|
|
addUrlFile relaxed url file = do
|
|
liftIO $ createDirectoryIfMissing True (parentDir file)
|
|
ifM (Annex.getState Annex.fast <||> pure relaxed)
|
|
( nodownload relaxed url file
|
|
, do
|
|
showAction $ "downloading " ++ url ++ " "
|
|
download url file
|
|
)
|
|
|
|
download :: String -> FilePath -> Annex Bool
|
|
download url file = do
|
|
dummykey <- genkey
|
|
tmp <- fromRepo $ gitAnnexTmpLocation dummykey
|
|
showOutput
|
|
ifM (runtransfer dummykey tmp)
|
|
( do
|
|
backend <- chooseBackend file
|
|
let source = KeySource
|
|
{ keyFilename = file
|
|
, contentLocation = tmp
|
|
, inodeCache = Nothing
|
|
}
|
|
k <- genKey source backend
|
|
case k of
|
|
Nothing -> return False
|
|
Just (key, _) -> cleanup url file key (Just tmp)
|
|
, return False
|
|
)
|
|
where
|
|
{- Generate a dummy key to use for this download, before we can
|
|
- examine the file and find its real key. This allows resuming
|
|
- downloads, as the dummy key for a given url is stable.
|
|
-
|
|
- If the assistant is running, actually hits the url here,
|
|
- to get the size, so it can display a pretty progress bar.
|
|
-}
|
|
genkey = do
|
|
pidfile <- fromRepo gitAnnexPidFile
|
|
size <- ifM (liftIO $ isJust <$> checkDaemon pidfile)
|
|
( do
|
|
headers <- getHttpHeaders
|
|
liftIO $ snd <$> Url.exists url headers
|
|
, return Nothing
|
|
)
|
|
Backend.URL.fromUrl url size
|
|
runtransfer dummykey tmp =
|
|
Transfer.download webUUID dummykey (Just file) Transfer.forwardRetry $ const $ do
|
|
liftIO $ createDirectoryIfMissing True (parentDir tmp)
|
|
downloadUrl [url] tmp
|
|
|
|
|
|
cleanup :: String -> FilePath -> Key -> Maybe FilePath -> Annex Bool
|
|
cleanup url file key mtmp = do
|
|
when (isJust mtmp) $
|
|
logStatus key InfoPresent
|
|
setUrlPresent key url
|
|
Command.Add.addLink file key False
|
|
whenM isDirect $ do
|
|
void $ addAssociatedFile key file
|
|
{- For moveAnnex to work in direct mode, the symlink
|
|
- must already exist, so flush the queue. -}
|
|
Annex.Queue.flush
|
|
maybe noop (moveAnnex key) mtmp
|
|
return True
|
|
|
|
nodownload :: Bool -> String -> FilePath -> Annex Bool
|
|
nodownload relaxed url file = do
|
|
headers <- getHttpHeaders
|
|
(exists, size) <- if relaxed
|
|
then pure (True, Nothing)
|
|
else liftIO $ Url.exists url headers
|
|
if exists
|
|
then do
|
|
key <- Backend.URL.fromUrl url size
|
|
cleanup url file key Nothing
|
|
else do
|
|
warning $ "unable to access url: " ++ url
|
|
return False
|
|
|
|
url2file :: URI -> Maybe Int -> Int -> FilePath
|
|
url2file url pathdepth pathmax = case pathdepth of
|
|
Nothing -> truncateFilePath pathmax $ escape fullurl
|
|
Just depth
|
|
| depth >= length urlbits -> frombits id
|
|
| depth > 0 -> frombits $ drop depth
|
|
| depth < 0 -> frombits $ reverse . take (negate depth) . reverse
|
|
| otherwise -> error "bad --pathdepth"
|
|
where
|
|
fullurl = uriRegName auth ++ uriPath url ++ uriQuery url
|
|
frombits a = intercalate "/" $ a urlbits
|
|
urlbits = map (truncateFilePath pathmax . escape) $ filter (not . null) $ split "/" fullurl
|
|
auth = fromMaybe (error $ "bad url " ++ show url) $ uriAuthority url
|
|
escape = replace "/" "_" . replace "?" "_"
|