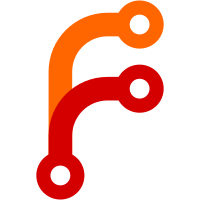
Leveraged the existing verification code by making it also check the retrievalSecurityPolicy. Also, prevented getViaTmp from running the download action at all when the retrievalSecurityPolicy is going to prevent verifying and so storing it. Added annex.security.allow-unverified-downloads. A per-remote version would be nice to have too, but would need more plumbing, so KISS. (Bill the Cat reference not too over the top I hope. The point is to make this something the user reads the documentation for before using.) A few calls to verifyKeyContent and getViaTmp, that don't involve downloads from remotes, have RetrievalAllKeysSecure hard-coded. It was also hard-coded for P2P.Annex and Command.RecvKey, to match the values of the corresponding remotes. A few things use retrieveKeyFile/retrieveKeyFileCheap without going through getViaTmp. * Command.Fsck when downloading content from a remote to verify it. That content does not get into the annex, so this is ok. * Command.AddUrl when using a remote to download an url; this is new content being added, so this is ok. This commit was sponsored by Fernando Jimenez on Patreon.
83 lines
2 KiB
Haskell
83 lines
2 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2011-2016 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.Reinject where
|
|
|
|
import Command
|
|
import Logs.Location
|
|
import Annex.Content
|
|
import Backend
|
|
import Types.KeySource
|
|
|
|
cmd :: Command
|
|
cmd = command "reinject" SectionUtility
|
|
"inject content of file back into annex"
|
|
(paramRepeating (paramPair "SRC" "DEST"))
|
|
(seek <$$> optParser)
|
|
|
|
data ReinjectOptions = ReinjectOptions
|
|
{ params :: CmdParams
|
|
, knownOpt :: Bool
|
|
}
|
|
|
|
optParser :: CmdParamsDesc -> Parser ReinjectOptions
|
|
optParser desc = ReinjectOptions
|
|
<$> cmdParams desc
|
|
<*> switch
|
|
( long "known"
|
|
<> help "inject all known files"
|
|
<> hidden
|
|
)
|
|
|
|
seek :: ReinjectOptions -> CommandSeek
|
|
seek os
|
|
| knownOpt os = withStrings startKnown (params os)
|
|
| otherwise = withWords startSrcDest (params os)
|
|
|
|
startSrcDest :: [FilePath] -> CommandStart
|
|
startSrcDest (src:dest:[])
|
|
| src == dest = stop
|
|
| otherwise = notAnnexed src $ do
|
|
showStart "reinject" dest
|
|
next $ ifAnnexed dest go stop
|
|
where
|
|
go key = ifM (verifyKeyContent RetrievalAllKeysSecure DefaultVerify UnVerified key src)
|
|
( perform src key
|
|
, error "failed"
|
|
)
|
|
startSrcDest _ = giveup "specify a src file and a dest file"
|
|
|
|
startKnown :: FilePath -> CommandStart
|
|
startKnown src = notAnnexed src $ do
|
|
showStart "reinject" src
|
|
mkb <- genKey (KeySource src src Nothing) Nothing
|
|
case mkb of
|
|
Nothing -> error "Failed to generate key"
|
|
Just (key, _) -> ifM (isKnownKey key)
|
|
( next $ perform src key
|
|
, do
|
|
warning "Not known content; skipping"
|
|
next $ next $ return True
|
|
)
|
|
|
|
notAnnexed :: FilePath -> CommandStart -> CommandStart
|
|
notAnnexed src = ifAnnexed src $
|
|
giveup $ "cannot used annexed file as src: " ++ src
|
|
|
|
perform :: FilePath -> Key -> CommandPerform
|
|
perform src key = ifM move
|
|
( next $ cleanup key
|
|
, error "failed"
|
|
)
|
|
where
|
|
move = checkDiskSpaceToGet key False $
|
|
moveAnnex key src
|
|
|
|
cleanup :: Key -> CommandCleanup
|
|
cleanup key = do
|
|
logStatus key InfoPresent
|
|
return True
|