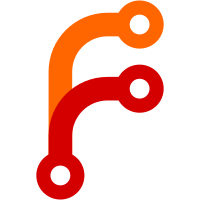
No behavior changes (hopefully), just adding SeekInput and plumbing it through to the JSON display code for later use. Over the course of 2 grueling days. withFilesNotInGit reimplemented in terms of seekHelper should be the only possible behavior change. It seems to test as behaving the same. Note that seekHelper dummies up the SeekInput in the case where segmentPaths' gives up on sorting the expanded paths because there are too many input paths. When SeekInput later gets exposed as a json field, that will result in it being a little bit wrong in the case where 100 or more paths are passed to a git-annex command. I think this is a subtle enough problem to not matter. If it does turn out to be a problem, fixing it would require splitting up the input parameters into groups of < 100, which would make git ls-files run perhaps more than is necessary. May want to revisit this, because that fix seems fairly low-impact.
84 lines
2.5 KiB
Haskell
84 lines
2.5 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2010 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.Copy where
|
|
|
|
import Command
|
|
import qualified Command.Move
|
|
import qualified Remote
|
|
import Annex.Wanted
|
|
import Annex.NumCopies
|
|
|
|
cmd :: Command
|
|
cmd = withGlobalOptions [jobsOption, jsonOptions, jsonProgressOption, annexedMatchingOptions] $
|
|
command "copy" SectionCommon
|
|
"copy content of files to/from another repository"
|
|
paramPaths (seek <--< optParser)
|
|
|
|
data CopyOptions = CopyOptions
|
|
{ copyFiles :: CmdParams
|
|
, fromToOptions :: FromToHereOptions
|
|
, keyOptions :: Maybe KeyOptions
|
|
, autoMode :: Bool
|
|
, batchOption :: BatchMode
|
|
}
|
|
|
|
optParser :: CmdParamsDesc -> Parser CopyOptions
|
|
optParser desc = CopyOptions
|
|
<$> cmdParams desc
|
|
<*> parseFromToHereOptions
|
|
<*> optional (parseKeyOptions <|> parseFailedTransfersOption)
|
|
<*> parseAutoOption
|
|
<*> parseBatchOption
|
|
|
|
instance DeferredParseClass CopyOptions where
|
|
finishParse v = CopyOptions
|
|
<$> pure (copyFiles v)
|
|
<*> finishParse (fromToOptions v)
|
|
<*> pure (keyOptions v)
|
|
<*> pure (autoMode v)
|
|
<*> pure (batchOption v)
|
|
|
|
seek :: CopyOptions -> CommandSeek
|
|
seek o = startConcurrency commandStages $ do
|
|
case batchOption o of
|
|
NoBatch -> withKeyOptions
|
|
(keyOptions o) (autoMode o) seeker
|
|
(commandAction . Command.Move.startKey (fromToOptions o) Command.Move.RemoveNever)
|
|
(withFilesInGitAnnex ww seeker)
|
|
=<< workTreeItems ww (copyFiles o)
|
|
Batch fmt -> batchAnnexedFilesMatching fmt seeker
|
|
where
|
|
ww = WarnUnmatchLsFiles
|
|
|
|
seeker = AnnexedFileSeeker
|
|
{ startAction = start o
|
|
, checkContentPresent = case fromToOptions o of
|
|
Right (FromRemote _) -> Just False
|
|
Right (ToRemote _) -> Just True
|
|
Left ToHere -> Just False
|
|
, usesLocationLog = True
|
|
}
|
|
|
|
{- A copy is just a move that does not delete the source file.
|
|
- However, auto mode avoids unnecessary copies, and avoids getting or
|
|
- sending non-preferred content. -}
|
|
start :: CopyOptions -> SeekInput -> RawFilePath -> Key -> CommandStart
|
|
start o si file key = stopUnless shouldCopy $
|
|
Command.Move.start (fromToOptions o) Command.Move.RemoveNever si file key
|
|
where
|
|
shouldCopy
|
|
| autoMode o = want <||> numCopiesCheck (fromRawFilePath file) key (<)
|
|
| otherwise = return True
|
|
want = case fromToOptions o of
|
|
Right (ToRemote dest) ->
|
|
(Remote.uuid <$> getParsed dest) >>= checkwantsend
|
|
Right (FromRemote _) -> checkwantget
|
|
Left ToHere -> checkwantget
|
|
|
|
checkwantsend = wantSend False (Just key) (AssociatedFile (Just file))
|
|
checkwantget = wantGet False (Just key) (AssociatedFile (Just file))
|