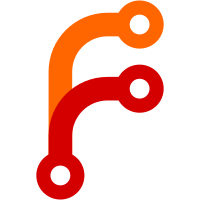
init: Fix a reversion in the last release that prevented automatically generating and setting a description for the repository. Seemed best to factor out uuidDescMapRaw that does not have the default mempty descrition behavior. I don't much like that behavior, but I know things depend on it. One thing in particular is `git annex info` which lists the uuids and descriptions; if the current repo has been initialized in some way that means it does not have a description, it would not show up w/o that. (Not only repos created due to this bug might lack that. For example a repo that was marked dead and had --drop-dead delete its git-annex branch info, and then came back from the dead would similarly not be in the uuid.log. Also there have been other versions of git-annex that didn't set a default description; for years there was no default description.)
61 lines
1.7 KiB
Haskell
61 lines
1.7 KiB
Haskell
{- git-annex uuid log
|
|
-
|
|
- uuid.log stores a list of known uuids, and their descriptions.
|
|
-
|
|
- Copyright 2010-2019 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Logs.UUID (
|
|
uuidLog,
|
|
describeUUID,
|
|
uuidDescMap,
|
|
uuidDescMapLoad,
|
|
uuidDescMapRaw,
|
|
) where
|
|
|
|
import Types.UUID
|
|
import Annex.Common
|
|
import Annex.VectorClock
|
|
import qualified Annex
|
|
import qualified Annex.Branch
|
|
import Logs
|
|
import Logs.UUIDBased
|
|
import qualified Annex.UUID
|
|
|
|
import qualified Data.Map.Strict as M
|
|
import qualified Data.ByteString.Lazy as L
|
|
import qualified Data.Attoparsec.ByteString.Lazy as A
|
|
|
|
{- Records a description for a uuid in the log. -}
|
|
describeUUID :: UUID -> UUIDDesc -> Annex ()
|
|
describeUUID uuid desc = do
|
|
c <- liftIO currentVectorClock
|
|
Annex.Branch.change uuidLog $
|
|
buildLogOld buildUUIDDesc . changeLog c uuid desc . parseUUIDLog
|
|
|
|
{- The map is cached for speed. -}
|
|
uuidDescMap :: Annex UUIDDescMap
|
|
uuidDescMap = maybe uuidDescMapLoad return =<< Annex.getState Annex.uuiddescmap
|
|
|
|
{- Read the uuidLog into a map, and cache it for later use.
|
|
-
|
|
- If the current repository has not been described, it is still included
|
|
- in the map with an empty description. -}
|
|
uuidDescMapLoad :: Annex UUIDDescMap
|
|
uuidDescMapLoad = do
|
|
m <- uuidDescMapRaw
|
|
u <- Annex.UUID.getUUID
|
|
let m' = M.insertWith preferold u mempty m
|
|
Annex.changeState $ \s -> s { Annex.uuiddescmap = Just m' }
|
|
return m'
|
|
where
|
|
preferold = flip const
|
|
|
|
{- Read the uuidLog into a map. Includes only actually set descriptions. -}
|
|
uuidDescMapRaw :: Annex UUIDDescMap
|
|
uuidDescMapRaw = simpleMap . parseUUIDLog <$> Annex.Branch.get uuidLog
|
|
|
|
parseUUIDLog :: L.ByteString -> Log UUIDDesc
|
|
parseUUIDLog = parseLogOld (UUIDDesc <$> A.takeByteString)
|