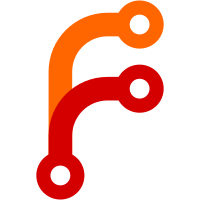
e53070c1f
quietly made it set the local git config too, but that was never
documented anywhere, and it had surprising results. If I set
annex.largefiles globally in a repo, I would expect to be able to change it
in another repo, and the original repo would get the change and use it,
rather than being stuck on the old value set there.
And, if I have a local annex.largefiles and set a different global default,
I'd be surprised to have my local setting overwritten.
annex.securehashesonly does need to be set locally, since it's a security
feature and the global is only a default until it gets set locally. So
special cased.
77 lines
1.9 KiB
Haskell
77 lines
1.9 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2017 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
{-# LANGUAGE OverloadedStrings #-}
|
|
|
|
module Command.Config where
|
|
|
|
import Command
|
|
import Logs.Config
|
|
import Config
|
|
import Git.Types (ConfigKey(..), fromConfigValue)
|
|
|
|
import qualified Data.ByteString.Char8 as S8
|
|
|
|
cmd :: Command
|
|
cmd = noMessages $ command "config" SectionSetup
|
|
"configuration stored in git-annex branch"
|
|
paramNothing (seek <$$> optParser)
|
|
|
|
data Action
|
|
= SetConfig ConfigKey ConfigValue
|
|
| GetConfig ConfigKey
|
|
| UnsetConfig ConfigKey
|
|
|
|
type Name = String
|
|
type Value = String
|
|
|
|
optParser :: CmdParamsDesc -> Parser Action
|
|
optParser _ = setconfig <|> getconfig <|> unsetconfig
|
|
where
|
|
setconfig = SetConfig
|
|
<$> strOption
|
|
( long "set"
|
|
<> help "set configuration"
|
|
<> metavar paramName
|
|
)
|
|
<*> strArgument
|
|
( metavar paramValue
|
|
)
|
|
getconfig = GetConfig <$> strOption
|
|
( long "get"
|
|
<> help "get configuration"
|
|
<> metavar paramName
|
|
)
|
|
unsetconfig = UnsetConfig <$> strOption
|
|
( long "unset"
|
|
<> help "unset configuration"
|
|
<> metavar paramName
|
|
)
|
|
|
|
seek :: Action -> CommandSeek
|
|
seek (SetConfig ck@(ConfigKey name) val) = commandAction $
|
|
startingUsualMessages (decodeBS' name) (ActionItemOther (Just (fromConfigValue val))) $ do
|
|
setGlobalConfig ck val
|
|
when (needLocalUpdate ck) $
|
|
setConfig ck (fromConfigValue val)
|
|
next $ return True
|
|
seek (UnsetConfig ck@(ConfigKey name)) = commandAction $
|
|
startingUsualMessages (decodeBS' name) (ActionItemOther (Just "unset")) $do
|
|
unsetGlobalConfig ck
|
|
when (needLocalUpdate ck) $
|
|
unsetConfig ck
|
|
next $ return True
|
|
seek (GetConfig ck) = commandAction $
|
|
startingCustomOutput (ActionItemOther Nothing) $ do
|
|
getGlobalConfig ck >>= \case
|
|
Nothing -> return ()
|
|
Just (ConfigValue v) -> liftIO $ S8.putStrLn v
|
|
next $ return True
|
|
|
|
needLocalUpdate :: ConfigKey -> Bool
|
|
needLocalUpdate (ConfigKey "annex.securehashesonly") = True
|
|
needLocalUpdate _ = False
|