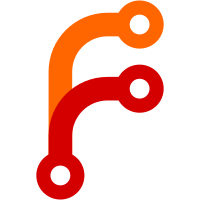
For simplicity, I've not tried to make it handle History yet, so when there is a history, a full import will still be done. Probably the right way to handle history is to first diff from the current tree to the last imported tree. Then, diff from the current tree to each of the historical trees, and recurse through the history diffing from child tree to parent tree. I don't think that will need a record of the previously imported historical trees, and so Logs.Import doesn't store them. Although I did leave room for future expansion in that log just in case. Next step will be to change importTree to importChanges and modify recordImportTree et all to handle it, by using adjustTree. Sponsored-by: Brett Eisenberg on Patreon
37 lines
1 KiB
Haskell
37 lines
1 KiB
Haskell
{- git-annex import logs
|
|
-
|
|
- Copyright 2023 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Logs.Import (
|
|
recordContentIdentifierTree,
|
|
getContentIdentifierTree
|
|
) where
|
|
|
|
import Annex.Common
|
|
import Git.Types
|
|
import Git.Sha
|
|
import Logs.File
|
|
|
|
import qualified Data.ByteString.Lazy as L
|
|
|
|
{- Records the sha of a tree that contains hashes of ContentIdentifiers
|
|
- that were imported from a remote. -}
|
|
recordContentIdentifierTree :: UUID -> Sha -> Annex ()
|
|
recordContentIdentifierTree u t = do
|
|
l <- calcRepo' (gitAnnexImportLog u)
|
|
writeLogFile l (fromRef t)
|
|
|
|
{- Gets the tree last recorded for a remote. -}
|
|
getContentIdentifierTree :: UUID -> Annex (Maybe Sha)
|
|
getContentIdentifierTree u = do
|
|
l <- calcRepo' (gitAnnexImportLog u)
|
|
-- This is safe because the log file is written atomically.
|
|
calcLogFileUnsafe l Nothing update
|
|
where
|
|
update l Nothing = extractSha (L.toStrict l)
|
|
-- Subsequent lines are ignored. This leaves room for future
|
|
-- expansion of what is logged.
|
|
update _l (Just l) = Just l
|