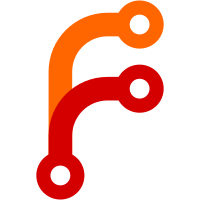
* addurl --preserve-filename: New option, uses server-provided filename without any sanitization, but with some security checking. Not yet implemented for remotes other than the web. * addurl, importfeed: Avoid adding filenames with leading '.', instead it will be replaced with '_'. This might be considered a security fix, but a CVE seems unwattanted. It was possible for addurl to create a dotfile, which could change behavior of some program. It was also possible for a web server to say the file name was ".git" or "foo/.git". That would not overrwrite the .git directory, but would cause addurl to fail; of course git won't add "foo/.git". sanitizeFilePath is too opinionated to remain in Utility, so moved it. The changes to mkSafeFilePath are because it used sanitizeFilePath. In particular: isDrive will never succeed, because "c:" gets munged to "c_" ".." gets sanitized now ".git" gets sanitized now It will never be null, because sanitizeFilePath keeps the length the same, and splitDirectories never returns a null path. Also, on the off chance a web server suggests a filename of "", ignore that, rather than trying to save to such a filename, which would fail in some way.
41 lines
1.1 KiB
Haskell
41 lines
1.1 KiB
Haskell
{- git-annex URL contents
|
|
-
|
|
- Copyright 2014 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Types.UrlContents (
|
|
UrlContents(..),
|
|
SafeFilePath,
|
|
mkSafeFilePath,
|
|
fromSafeFilePath
|
|
) where
|
|
|
|
import Utility.Url
|
|
import Annex.UntrustedFilePath
|
|
|
|
import System.FilePath
|
|
|
|
data UrlContents
|
|
-- An URL contains a file, whose size may be known.
|
|
-- There might be a nicer filename to use.
|
|
= UrlContents (Maybe Integer) (Maybe SafeFilePath)
|
|
-- Sometimes an URL points to multiple files, each accessible
|
|
-- by their own URL.
|
|
| UrlMulti [(URLString, Maybe Integer, SafeFilePath)]
|
|
|
|
-- This is a FilePath, from an untrusted source,
|
|
-- sanitized so it doesn't contain any directory traversal tricks
|
|
-- and is always relative. It can still contain subdirectories.
|
|
-- Any unusual characters are also filtered out.
|
|
newtype SafeFilePath = SafeFilePath FilePath
|
|
deriving (Show)
|
|
|
|
mkSafeFilePath :: FilePath -> SafeFilePath
|
|
mkSafeFilePath p = SafeFilePath $ if null p' then "file" else p'
|
|
where
|
|
p' = joinPath $ map sanitizeFilePath $ splitDirectories p
|
|
|
|
fromSafeFilePath :: SafeFilePath -> FilePath
|
|
fromSafeFilePath (SafeFilePath p) = p
|