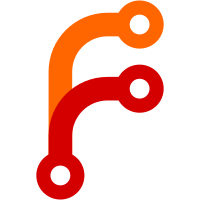
(And a vpop command, which is still a bit buggy.) Still need to do vadd and vrm, though this also adds their documentation. Currently not very happy with the view log data serialization. I had to lose the TDFA regexps temporarily, so I can have Read/Show instances of View. I expect the view log format will change in some incompatable way later, probably adding last known refs for the parent branch to View or something like that. Anyway, it basically works, although it's a bit slow looking up the metadata. The actual git branch construction is about as fast as it can be using the current git plumbing. This commit was sponsored by Peter Hogg.
54 lines
1.4 KiB
Haskell
54 lines
1.4 KiB
Haskell
{- types for metadata based branch views
|
|
-
|
|
- Copyright 2014 Joey Hess <joey@kitenet.net>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Types.View where
|
|
|
|
import Common.Annex
|
|
import Types.MetaData
|
|
import Utility.QuickCheck
|
|
import qualified Git
|
|
|
|
import qualified Data.Set as S
|
|
|
|
{- A view is a list of fields with filters on their allowed values,
|
|
- which are applied to files in a parent git branch. -}
|
|
data View = View
|
|
{ viewParentBranch :: Git.Branch
|
|
, viewComponents :: [ViewComponent]
|
|
}
|
|
deriving (Eq, Show)
|
|
|
|
instance Arbitrary View where
|
|
arbitrary = View <$> pure (Git.Ref "master") <*> arbitrary
|
|
|
|
data ViewComponent = ViewComponent
|
|
{ viewField :: MetaField
|
|
, viewFilter :: ViewFilter
|
|
}
|
|
deriving (Eq, Show, Read)
|
|
|
|
instance Arbitrary ViewComponent where
|
|
arbitrary = ViewComponent <$> arbitrary <*> arbitrary
|
|
|
|
{- Only files with metadata matching the view are displayed. -}
|
|
type FileView = FilePath
|
|
type MkFileView = FilePath -> FileView
|
|
|
|
data ViewFilter
|
|
= FilterValues (S.Set MetaValue)
|
|
| FilterGlob String
|
|
deriving (Eq, Show, Read)
|
|
|
|
instance Arbitrary ViewFilter where
|
|
arbitrary = do
|
|
size <- arbitrarySizedBoundedIntegral `suchThat` (< 100)
|
|
FilterValues . S.fromList <$> vector size
|
|
|
|
{- Can a ViewFilter match multiple different MetaValues? -}
|
|
multiValue :: ViewFilter -> Bool
|
|
multiValue (FilterValues s) = S.size s > 1
|
|
multiValue (FilterGlob _) = True
|