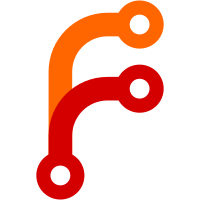
Avoid threads emitting json at the same time and scrambling, which was still possible even with the buffering, just less likely. Converted json IO actions to JSONChunk data too.
49 lines
1.1 KiB
Haskell
49 lines
1.1 KiB
Haskell
{- git-annex Messages data types
|
|
-
|
|
- Copyright 2012 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
{-# LANGUAGE CPP #-}
|
|
|
|
module Types.Messages where
|
|
|
|
import Data.Default
|
|
import qualified Data.ByteString.Lazy as B
|
|
|
|
#ifdef WITH_CONCURRENTOUTPUT
|
|
import System.Console.Regions (ConsoleRegion)
|
|
#endif
|
|
|
|
data OutputType = NormalOutput | QuietOutput | JSONOutput Bool
|
|
deriving (Show)
|
|
|
|
data SideActionBlock = NoBlock | StartBlock | InBlock
|
|
deriving (Eq)
|
|
|
|
data MessageState = MessageState
|
|
{ outputType :: OutputType
|
|
, concurrentOutputEnabled :: Bool
|
|
, sideActionBlock :: SideActionBlock
|
|
, implicitMessages :: Bool
|
|
#ifdef WITH_CONCURRENTOUTPUT
|
|
, consoleRegion :: Maybe ConsoleRegion
|
|
, consoleRegionErrFlag :: Bool
|
|
#endif
|
|
, jsonBuffer :: B.ByteString
|
|
}
|
|
|
|
instance Default MessageState
|
|
where
|
|
def = MessageState
|
|
{ outputType = NormalOutput
|
|
, concurrentOutputEnabled = False
|
|
, sideActionBlock = NoBlock
|
|
, implicitMessages = True
|
|
#ifdef WITH_CONCURRENTOUTPUT
|
|
, consoleRegion = Nothing
|
|
, consoleRegionErrFlag = False
|
|
#endif
|
|
, jsonBuffer = B.empty
|
|
}
|