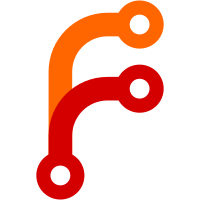
This preserves the workaround for the old bug that caused NoUUID items to be stored in the log, prefixing log lines with " ". It's now handled implicitly, by using takeWhile1 (/= ' ') to get the uuid. There is a behavior change from the old parser, which split the value into words and then recombined it. That meant that "foo bar" and "foo\tbar" came out as "foo bar". That behavior was not documented, and seems surprising; it meant that after a git-annex describe here "foo bar", you wouldn't get that same string back out when git-annex displayed repo descriptions. Otoh, some other parsers relied on the old behavior, and the attoparsec rewrites had to deal with the issue themselves... For group.log, there are some edge cases around the user providing a group name with a leading or trailing space. The old parser would ignore such excess whitespace. The new parser does too, because the alternative is to refuse to parse something like " group1 group2 " due to excess whitespace, which would be even more confusing behavior. The only git-annex branch log file that is not converted to attoparsec and bytestring-builder now is transitions.log.
49 lines
1.4 KiB
Haskell
49 lines
1.4 KiB
Haskell
{- git-annex trust log, pure operations
|
|
-
|
|
- Copyright 2010-2018 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
{-# LANGUAGE OverloadedStrings #-}
|
|
|
|
module Logs.Trust.Pure where
|
|
|
|
import Annex.Common
|
|
import Types.TrustLevel
|
|
import Logs.UUIDBased
|
|
|
|
import qualified Data.ByteString.Lazy as L
|
|
import qualified Data.Attoparsec.ByteString.Lazy as A
|
|
import qualified Data.Attoparsec.ByteString.Char8 as A8
|
|
import Data.ByteString.Builder
|
|
|
|
calcTrustMap :: L.ByteString -> TrustMap
|
|
calcTrustMap = simpleMap . parseLog trustLevelParser
|
|
|
|
trustLevelParser :: A.Parser TrustLevel
|
|
trustLevelParser = (totrust <$> A8.anyChar <* A.endOfInput)
|
|
-- The trust log used to only list trusted repos, without a
|
|
-- value for the trust status
|
|
<|> (const Trusted <$> A.endOfInput)
|
|
where
|
|
totrust '1' = Trusted
|
|
totrust '0' = UnTrusted
|
|
totrust 'X' = DeadTrusted
|
|
-- Allow for future expansion by treating unknown trust levels as
|
|
-- semitrusted.
|
|
totrust _ = SemiTrusted
|
|
|
|
buildTrustLevel :: TrustLevel -> Builder
|
|
buildTrustLevel Trusted = byteString "1"
|
|
buildTrustLevel UnTrusted = byteString "0"
|
|
buildTrustLevel DeadTrusted = byteString "X"
|
|
buildTrustLevel SemiTrusted = byteString "?"
|
|
|
|
prop_parse_build_TrustLevelLog :: Bool
|
|
prop_parse_build_TrustLevelLog = all check [minBound .. maxBound]
|
|
where
|
|
check l =
|
|
let v = A.parseOnly trustLevelParser $ L.toStrict $
|
|
toLazyByteString $ buildTrustLevel l
|
|
in v == Right l
|