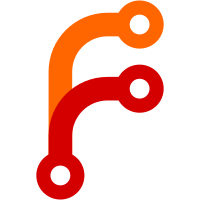
This preserves the workaround for the old bug that caused NoUUID items to be stored in the log, prefixing log lines with " ". It's now handled implicitly, by using takeWhile1 (/= ' ') to get the uuid. There is a behavior change from the old parser, which split the value into words and then recombined it. That meant that "foo bar" and "foo\tbar" came out as "foo bar". That behavior was not documented, and seems surprising; it meant that after a git-annex describe here "foo bar", you wouldn't get that same string back out when git-annex displayed repo descriptions. Otoh, some other parsers relied on the old behavior, and the attoparsec rewrites had to deal with the issue themselves... For group.log, there are some edge cases around the user providing a group name with a leading or trailing space. The old parser would ignore such excess whitespace. The new parser does too, because the alternative is to refuse to parse something like " group1 group2 " due to excess whitespace, which would be even more confusing behavior. The only git-annex branch log file that is not converted to attoparsec and bytestring-builder now is transitions.log.
82 lines
2.9 KiB
Haskell
82 lines
2.9 KiB
Haskell
{- unparsed preferred content expressions
|
|
-
|
|
- Copyright 2012-2019 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Logs.PreferredContent.Raw where
|
|
|
|
import Annex.Common
|
|
import qualified Annex.Branch
|
|
import qualified Annex
|
|
import Logs
|
|
import Logs.UUIDBased
|
|
import Logs.MapLog
|
|
import Types.StandardGroups
|
|
import Types.Group
|
|
|
|
import qualified Data.Map as M
|
|
import qualified Data.ByteString.Lazy as L
|
|
import qualified Data.Attoparsec.ByteString.Lazy as A
|
|
import Data.ByteString.Builder
|
|
|
|
{- Changes the preferred content configuration of a remote. -}
|
|
preferredContentSet :: UUID -> PreferredContentExpression -> Annex ()
|
|
preferredContentSet = setLog preferredContentLog
|
|
|
|
requiredContentSet :: UUID -> PreferredContentExpression -> Annex ()
|
|
requiredContentSet = setLog requiredContentLog
|
|
|
|
setLog :: FilePath -> UUID -> PreferredContentExpression -> Annex ()
|
|
setLog logfile uuid@(UUID _) val = do
|
|
c <- liftIO currentVectorClock
|
|
Annex.Branch.change logfile $
|
|
buildLog buildPreferredContentExpression
|
|
. changeLog c uuid val
|
|
. parseLog parsePreferredContentExpression
|
|
Annex.changeState $ \s -> s
|
|
{ Annex.preferredcontentmap = Nothing
|
|
, Annex.requiredcontentmap = Nothing
|
|
}
|
|
setLog _ NoUUID _ = error "unknown UUID; cannot modify"
|
|
|
|
{- Changes the preferred content configuration of a group. -}
|
|
groupPreferredContentSet :: Group -> PreferredContentExpression -> Annex ()
|
|
groupPreferredContentSet g val = do
|
|
c <- liftIO currentVectorClock
|
|
Annex.Branch.change groupPreferredContentLog $
|
|
buildGroupPreferredContent
|
|
. changeMapLog c g val
|
|
. parseGroupPreferredContent
|
|
Annex.changeState $ \s -> s { Annex.preferredcontentmap = Nothing }
|
|
|
|
parseGroupPreferredContent :: L.ByteString -> MapLog Group String
|
|
parseGroupPreferredContent = parseMapLog parsegroup parsestring
|
|
where
|
|
parsegroup = Group <$> A.takeByteString
|
|
parsestring = decodeBS <$> A.takeByteString
|
|
|
|
buildGroupPreferredContent :: MapLog Group PreferredContentExpression -> Builder
|
|
buildGroupPreferredContent = buildMapLog buildgroup buildexpr
|
|
where
|
|
buildgroup (Group g) = byteString g
|
|
buildexpr = byteString . encodeBS
|
|
|
|
parsePreferredContentExpression :: A.Parser PreferredContentExpression
|
|
parsePreferredContentExpression = decodeBS <$> A.takeByteString
|
|
|
|
buildPreferredContentExpression :: PreferredContentExpression -> Builder
|
|
buildPreferredContentExpression = byteString . encodeBS
|
|
|
|
preferredContentMapRaw :: Annex (M.Map UUID PreferredContentExpression)
|
|
preferredContentMapRaw = simpleMap . parseLog parsePreferredContentExpression
|
|
<$> Annex.Branch.get preferredContentLog
|
|
|
|
requiredContentMapRaw :: Annex (M.Map UUID PreferredContentExpression)
|
|
requiredContentMapRaw = simpleMap . parseLog parsePreferredContentExpression
|
|
<$> Annex.Branch.get requiredContentLog
|
|
|
|
groupPreferredContentMapRaw :: Annex (M.Map Group PreferredContentExpression)
|
|
groupPreferredContentMapRaw = simpleMap . parseGroupPreferredContent
|
|
<$> Annex.Branch.get groupPreferredContentLog
|