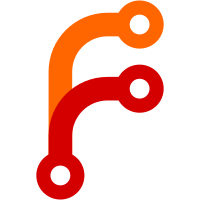
This is to avoid inserting a cluster uuid into the location log when only dead nodes in the cluster contain the content of a key. One reason why this is necessary is Remote.keyLocations, which excludes dead repositories from the list. But there are probably many more. Implementing this was challenging, because Logs.Location importing Logs.Cluster which imports Logs.Trust which imports Remote.List resulted in an import cycle through several other modules. Resorted to making Logs.Location not import Logs.Cluster, and instead it assumes that Annex.clusters gets populated when necessary before it's called. That's done in Annex.Startup, which is run by the git-annex command (but not other commands) at early startup in initialized repos. Or, is run after initialization. Note that is Remote.Git, it is unable to import Annex.Startup, because Remote.Git importing Logs.Cluster leads the the same import cycle. So ensureInitialized is not passed annexStartup in there. Other commands, like git-annex-shell currently don't run annexStartup either. So there are cases where Logs.Location will not see clusters. So it won't add any cluster UUIDs when loading the log. That's ok, the only reason to do that is to make display of where objects are located include clusters, and to make commands like git-annex get --from treat keys as being located in a cluster. git-annex-shell certainly does not do anything like that, and I'm pretty sure Remote.Git (and callers to Remote.Git.onLocalRepo) don't either.
54 lines
1.4 KiB
Haskell
54 lines
1.4 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2010-2014 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.ConfigList where
|
|
|
|
import Command
|
|
import Annex.UUID
|
|
import Annex.Init
|
|
import qualified Annex.Branch
|
|
import qualified Git.Config
|
|
import Git.Types
|
|
import Remote.GCrypt (coreGCryptId)
|
|
import qualified CmdLine.GitAnnexShell.Fields as Fields
|
|
import CmdLine.GitAnnexShell.Checks
|
|
import Annex.Startup
|
|
|
|
cmd :: Command
|
|
cmd = noCommit $ dontCheck repoExists $
|
|
command "configlist" SectionPlumbing
|
|
"outputs relevant git configuration"
|
|
paramNothing (withParams seek)
|
|
|
|
seek :: CmdParams -> CommandSeek
|
|
seek = withNothing (commandAction start)
|
|
|
|
start :: CommandStart
|
|
start = do
|
|
u <- findOrGenUUID
|
|
showConfig configkeyUUID $ fromUUID u
|
|
showConfig coreGCryptId . fromConfigValue
|
|
=<< fromRepo (Git.Config.get coreGCryptId mempty)
|
|
stop
|
|
where
|
|
showConfig k v = liftIO $ putStrLn $ fromConfigKey k ++ "=" ++ v
|
|
|
|
{- The repository may not yet have a UUID; automatically initialize it
|
|
- when there's a git-annex branch available or if the autoinit field was
|
|
- set. -}
|
|
findOrGenUUID :: Annex UUID
|
|
findOrGenUUID = do
|
|
u <- getUUID
|
|
if u /= NoUUID
|
|
then return u
|
|
else ifM (Annex.Branch.hasSibling <||> (isJust <$> Fields.getField Fields.autoInit))
|
|
( do
|
|
liftIO checkNotReadOnly
|
|
initialize startupAnnex Nothing Nothing
|
|
getUUID
|
|
, return NoUUID
|
|
)
|