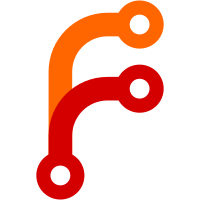
This does not change the overall license of the git-annex program, which was already AGPL due to a number of sources files being AGPL already. Legally speaking, I'm adding a new license under which these files are now available; I already released their current contents under the GPL license. Now they're dual licensed GPL and AGPL. However, I intend for all my future changes to these files to only be released under the AGPL license, and I won't be tracking the dual licensing status, so I'm simply changing the license statement to say it's AGPL. (In some cases, others wrote parts of the code of a file and released it under the GPL; but in all cases I have contributed a significant portion of the code in each file and it's that code that is getting the AGPL license; the GPL license of other contributors allows combining with AGPL code.)
51 lines
1.2 KiB
Haskell
51 lines
1.2 KiB
Haskell
{- git-annex trust levels
|
|
-
|
|
- Copyright 2010 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
{-# LANGUAGE FlexibleInstances #-}
|
|
|
|
module Types.TrustLevel (
|
|
TrustLevel(..),
|
|
TrustMap,
|
|
readTrustLevel,
|
|
showTrustLevel,
|
|
prop_read_show_TrustLevel
|
|
) where
|
|
|
|
import qualified Data.Map as M
|
|
import Data.Default
|
|
import Data.Ord
|
|
|
|
import Types.UUID
|
|
|
|
data TrustLevel = DeadTrusted | UnTrusted | SemiTrusted | Trusted
|
|
deriving (Eq, Enum, Ord, Bounded, Show)
|
|
|
|
instance Default TrustLevel where
|
|
def = SemiTrusted
|
|
|
|
instance Default (Down TrustLevel) where
|
|
def = Down def
|
|
|
|
type TrustMap = M.Map UUID TrustLevel
|
|
|
|
readTrustLevel :: String -> Maybe TrustLevel
|
|
readTrustLevel "trusted" = Just Trusted
|
|
readTrustLevel "untrusted" = Just UnTrusted
|
|
readTrustLevel "semitrusted" = Just SemiTrusted
|
|
readTrustLevel "dead" = Just DeadTrusted
|
|
readTrustLevel _ = Nothing
|
|
|
|
showTrustLevel :: TrustLevel -> String
|
|
showTrustLevel Trusted = "trusted"
|
|
showTrustLevel UnTrusted = "untrusted"
|
|
showTrustLevel SemiTrusted = "semitrusted"
|
|
showTrustLevel DeadTrusted = "dead"
|
|
|
|
prop_read_show_TrustLevel :: Bool
|
|
prop_read_show_TrustLevel = all check [minBound .. maxBound]
|
|
where
|
|
check l = readTrustLevel (showTrustLevel l) == Just l
|