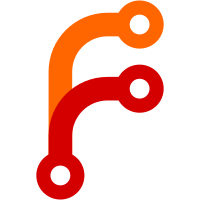
Work around git cat-file --batch's protocol not supporting newlines by running git cat-file not batched and passing the filename as a parameter. Of course this is quite a lot less efficient, especially because it currently runs it multiple times to query for different pieces of information. Also, it has subtly different behavior when the batch process was started and then some changes were made, in which case the batch process sees the old index but this workaround sees the current index. Since that batch behavior is mostly a problem that affects the assistant and has to be worked around in it, I think I can get away with this difference. I don't know of any other problems with newlines in filenames, everything else in git I can think of supports -z. And git-annex's json output supports newlines in filenames so downstream parsers from git-annex will be ok. git-annex commands that use --batch themselves don't support newlines in input filenames; using --json --batch is currently a way around that problem. This commit was sponsored by Ewen McNeill on Patreon.
67 lines
1.6 KiB
Haskell
67 lines
1.6 KiB
Haskell
{- Parts of the Prelude are partial functions, which are a common source of
|
|
- bugs.
|
|
-
|
|
- This exports functions that conflict with the prelude, which avoids
|
|
- them being accidentally used.
|
|
-}
|
|
|
|
{-# OPTIONS_GHC -fno-warn-tabs #-}
|
|
|
|
module Utility.PartialPrelude where
|
|
|
|
import qualified Data.Maybe
|
|
|
|
{- read should be avoided, as it throws an error
|
|
- Instead, use: readish -}
|
|
read :: Read a => String -> a
|
|
read = Prelude.read
|
|
|
|
{- head is a partial function; head [] is an error
|
|
- Instead, use: take 1 or headMaybe -}
|
|
head :: [a] -> a
|
|
head = Prelude.head
|
|
|
|
{- tail is also partial
|
|
- Instead, use: drop 1 -}
|
|
tail :: [a] -> [a]
|
|
tail = Prelude.tail
|
|
|
|
{- init too
|
|
- Instead, use: beginning -}
|
|
init :: [a] -> [a]
|
|
init = Prelude.init
|
|
|
|
{- last too
|
|
- Instead, use: end or lastMaybe -}
|
|
last :: [a] -> a
|
|
last = Prelude.last
|
|
|
|
{- Attempts to read a value from a String.
|
|
-
|
|
- Unlike Text.Read.readMaybe, this ignores leading/trailing whitespace,
|
|
- and throws away any trailing text after the part that can be read.
|
|
-}
|
|
readish :: Read a => String -> Maybe a
|
|
readish s = case reads s of
|
|
((x,_):_) -> Just x
|
|
_ -> Nothing
|
|
|
|
{- Like head but Nothing on empty list. -}
|
|
headMaybe :: [a] -> Maybe a
|
|
headMaybe = Data.Maybe.listToMaybe
|
|
|
|
{- Like last but Nothing on empty list. -}
|
|
lastMaybe :: [a] -> Maybe a
|
|
lastMaybe [] = Nothing
|
|
lastMaybe v = Just $ Prelude.last v
|
|
|
|
{- All but the last element of a list.
|
|
- (Like init, but no error on an empty list.) -}
|
|
beginning :: [a] -> [a]
|
|
beginning [] = []
|
|
beginning l = Prelude.init l
|
|
|
|
{- Like last, but no error on an empty list. -}
|
|
end :: [a] -> [a]
|
|
end [] = []
|
|
end l = [Prelude.last l]
|