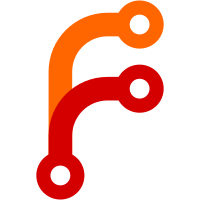
Now `git annex info $remote` shows info specific to the type of the remote, for example, it shows the rsync url. Remote types that support encryption or chunking also include that in their info. This commit was sponsored by Ævar Arnfjörð Bjarmason.
37 lines
1,018 B
Haskell
37 lines
1,018 B
Haskell
{- Utilities for git remotes.
|
|
-
|
|
- Copyright 2011-2014 Joey Hess <joey@kitenet.net>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Remote.Helper.Git where
|
|
|
|
import Common.Annex
|
|
import qualified Git
|
|
import Types.Availability
|
|
|
|
repoCheap :: Git.Repo -> Bool
|
|
repoCheap = not . Git.repoIsUrl
|
|
|
|
localpathCalc :: Git.Repo -> Maybe FilePath
|
|
localpathCalc r
|
|
| availabilityCalc r == GloballyAvailable = Nothing
|
|
| otherwise = Just $ Git.repoPath r
|
|
|
|
availabilityCalc :: Git.Repo -> Availability
|
|
availabilityCalc r
|
|
| (Git.repoIsLocal r || Git.repoIsLocalUnknown r) = LocallyAvailable
|
|
| otherwise = GloballyAvailable
|
|
|
|
{- Avoids performing an action on a local repository that's not usable.
|
|
- Does not check that the repository is still available on disk. -}
|
|
guardUsable :: Git.Repo -> Annex a -> Annex a -> Annex a
|
|
guardUsable r fallback a
|
|
| Git.repoIsLocalUnknown r = fallback
|
|
| otherwise = a
|
|
|
|
gitRepoInfo :: Git.Repo -> [(String, String)]
|
|
gitRepoInfo r =
|
|
[ ("repository location", Git.repoLocation r)
|
|
]
|