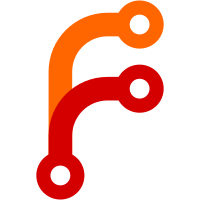
This needs the content to be present in order to hash it. But it's not possible for a module used by Backend.URL to call inAnnex because that would entail a dependency loop. So instead, rely on the fact that Command.Migrate calls inAnnex before performing a migration. But, Command.ExamineKey calls fastMigrate and the key may or may not exist, and it's not wanting to actually perform a migration in any case. To handle that, had to add an additional value to fastMigrate to indicate whether the content is inAnnex. Factored generateEquivilantKey out of Remote.Web. Note that migrateFromURLToVURL hardcodes use of the SHA256E backend. It would have been difficult not to, given all the dependency loop issues. But --backend and annex.backend are used to tell git-annex migrate to use VURL in any case, so there's no config knob that the user could expect to configure that. Sponsored-by: Brock Spratlen on Patreon
43 lines
1.1 KiB
Haskell
43 lines
1.1 KiB
Haskell
{- git-annex URL backend -- keys whose content is available from urls.
|
|
-
|
|
- Copyright 2011-2024 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Backend.URL (
|
|
backends,
|
|
fromUrl
|
|
) where
|
|
|
|
import Annex.Common
|
|
import Types.Key
|
|
import Types.Backend
|
|
import Backend.Utilities
|
|
import Backend.VURL.Utilities (migrateFromURLToVURL)
|
|
|
|
backends :: [Backend]
|
|
backends = [backendURL]
|
|
|
|
backendURL :: Backend
|
|
backendURL = Backend
|
|
{ backendVariety = URLKey
|
|
, genKey = Nothing
|
|
, verifyKeyContent = Nothing
|
|
, verifyKeyContentIncrementally = Nothing
|
|
, canUpgradeKey = Nothing
|
|
, fastMigrate = Just migrateFromURLToVURL
|
|
-- The content of an url can change at any time, so URL keys are
|
|
-- not stable.
|
|
, isStableKey = const False
|
|
, isCryptographicallySecure = False
|
|
, isCryptographicallySecureKey = const (pure False)
|
|
}
|
|
|
|
{- Every unique url has a corresponding key. -}
|
|
fromUrl :: String -> Maybe Integer -> Bool -> Key
|
|
fromUrl url size verifiable = mkKey $ \k -> k
|
|
{ keyName = genKeyName url
|
|
, keyVariety = if verifiable then VURLKey else URLKey
|
|
, keySize = size
|
|
}
|