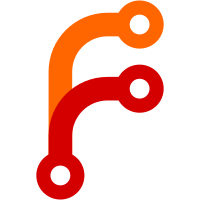
This does not change the overall license of the git-annex program, which was already AGPL due to a number of sources files being AGPL already. Legally speaking, I'm adding a new license under which these files are now available; I already released their current contents under the GPL license. Now they're dual licensed GPL and AGPL. However, I intend for all my future changes to these files to only be released under the AGPL license, and I won't be tracking the dual licensing status, so I'm simply changing the license statement to say it's AGPL. (In some cases, others wrote parts of the code of a file and released it under the GPL; but in all cases I have contributed a significant portion of the code in each file and it's that code that is getting the AGPL license; the GPL license of other contributors allows combining with AGPL code.)
71 lines
2.1 KiB
Haskell
71 lines
2.1 KiB
Haskell
{- git-annex presence log
|
|
-
|
|
- This is used to store presence information in the git-annex branch in
|
|
- a way that can be union merged.
|
|
-
|
|
- A line of the log will look like: "date N INFO"
|
|
- Where N=1 when the INFO is present, 0 otherwise.
|
|
-
|
|
- Copyright 2010-2014 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Logs.Presence (
|
|
module X,
|
|
addLog,
|
|
maybeAddLog,
|
|
readLog,
|
|
logNow,
|
|
currentLog,
|
|
currentLogInfo,
|
|
historicalLogInfo,
|
|
) where
|
|
|
|
import Logs.Presence.Pure as X
|
|
import Annex.Common
|
|
import Annex.VectorClock
|
|
import qualified Annex.Branch
|
|
import Git.Types (RefDate)
|
|
|
|
{- Adds a LogLine to the log, removing any LogLines that are obsoleted by
|
|
- adding it. -}
|
|
addLog :: FilePath -> LogLine -> Annex ()
|
|
addLog file line = Annex.Branch.change file $ \b ->
|
|
buildLog $ compactLog (line : parseLog b)
|
|
|
|
{- When a LogLine already exists with the same status and info, but an
|
|
- older timestamp, that LogLine is preserved, rather than updating the log
|
|
- with a newer timestamp.
|
|
-}
|
|
maybeAddLog :: FilePath -> LogLine -> Annex ()
|
|
maybeAddLog file line = Annex.Branch.maybeChange file $ \s -> do
|
|
m <- insertNewStatus line $ logMap $ parseLog s
|
|
return $ buildLog $ mapLog m
|
|
|
|
{- Reads a log file.
|
|
- Note that the LogLines returned may be in any order. -}
|
|
readLog :: FilePath -> Annex [LogLine]
|
|
readLog = parseLog <$$> Annex.Branch.get
|
|
|
|
{- Generates a new LogLine with the current time. -}
|
|
logNow :: LogStatus -> LogInfo -> Annex LogLine
|
|
logNow s i = do
|
|
c <- liftIO currentVectorClock
|
|
return $ LogLine c s i
|
|
|
|
{- Reads a log and returns only the info that is still in effect. -}
|
|
currentLogInfo :: FilePath -> Annex [LogInfo]
|
|
currentLogInfo file = map info <$> currentLog file
|
|
|
|
currentLog :: FilePath -> Annex [LogLine]
|
|
currentLog file = filterPresent <$> readLog file
|
|
|
|
{- Reads a historical version of a log and returns the info that was in
|
|
- effect at that time.
|
|
-
|
|
- The date is formatted as shown in gitrevisions man page.
|
|
-}
|
|
historicalLogInfo :: RefDate -> FilePath -> Annex [LogInfo]
|
|
historicalLogInfo refdate file = map info . filterPresent . parseLog
|
|
<$> Annex.Branch.getHistorical refdate file
|