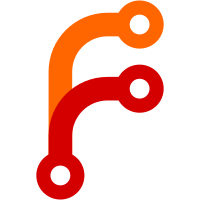
When a file is changed in direct mode, the old content is probably lost (at least from the local repo), and bookeeping needs to be updated to reflect this. Also, synthetic add events are generated at assistant startup, so make it detect when the file has not really changed, and avoid re-adding it. This does add the overhead of querying the runing git cat-file for the key that's recorded in git for the file, each time a file is added or modified in direct mode.
49 lines
1.3 KiB
Haskell
49 lines
1.3 KiB
Haskell
{- git cat-file interface, with handle automatically stored in the Annex monad
|
|
-
|
|
- Copyright 2011 Joey Hess <joey@kitenet.net>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Annex.CatFile (
|
|
catFile,
|
|
catObject,
|
|
catObjectDetails,
|
|
catKey,
|
|
catFileHandle
|
|
) where
|
|
|
|
import qualified Data.ByteString.Lazy as L
|
|
|
|
import Common.Annex
|
|
import qualified Git
|
|
import qualified Git.CatFile
|
|
import qualified Annex
|
|
import Git.Types
|
|
|
|
catFile :: Git.Branch -> FilePath -> Annex L.ByteString
|
|
catFile branch file = do
|
|
h <- catFileHandle
|
|
liftIO $ Git.CatFile.catFile h branch file
|
|
|
|
catObject :: Git.Ref -> Annex L.ByteString
|
|
catObject ref = do
|
|
h <- catFileHandle
|
|
liftIO $ Git.CatFile.catObject h ref
|
|
|
|
catObjectDetails :: Git.Ref -> Annex (Maybe (L.ByteString, Sha))
|
|
catObjectDetails ref = do
|
|
h <- catFileHandle
|
|
liftIO $ Git.CatFile.catObjectDetails h ref
|
|
|
|
catFileHandle :: Annex Git.CatFile.CatFileHandle
|
|
catFileHandle = maybe startup return =<< Annex.getState Annex.catfilehandle
|
|
where
|
|
startup = do
|
|
h <- inRepo Git.CatFile.catFileStart
|
|
Annex.changeState $ \s -> s { Annex.catfilehandle = Just h }
|
|
return h
|
|
|
|
{- From the Sha or Ref of a symlink back to the key. -}
|
|
catKey :: Ref -> Annex (Maybe Key)
|
|
catKey ref = fileKey . takeFileName . encodeW8 . L.unpack <$> catObject ref
|