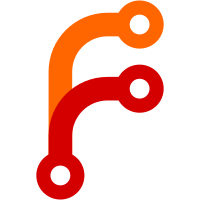
So, I have a type checked safe handling of filenames starting with dashes, throughout the code.
47 lines
1.3 KiB
Haskell
47 lines
1.3 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2010 Joey Hess <joey@kitenet.net>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.PreCommit where
|
|
|
|
import Control.Monad.State (liftIO)
|
|
|
|
import Command
|
|
import qualified Annex
|
|
import qualified GitRepo as Git
|
|
import qualified Command.Add
|
|
import qualified Command.Fix
|
|
import Messages
|
|
import Utility
|
|
|
|
command :: [Command]
|
|
command = [Command "pre-commit" paramPath seek "run by git pre-commit hook"]
|
|
|
|
{- The pre-commit hook needs to fix symlinks to all files being committed.
|
|
- And, it needs to inject unlocked files into the annex. -}
|
|
seek :: [CommandSeek]
|
|
seek = [withFilesToBeCommitted Command.Fix.start,
|
|
withFilesUnlockedToBeCommitted start]
|
|
|
|
start :: CommandStartBackendFile
|
|
start pair = return $ Just $ perform pair
|
|
|
|
perform :: BackendFile -> CommandPerform
|
|
perform pair@(file, _) = do
|
|
ok <- doCommand $ Command.Add.start pair
|
|
if ok
|
|
then return $ Just $ cleanup file
|
|
else error $ "failed to add " ++ filePathToString file ++ "; canceling commit"
|
|
|
|
cleanup :: FilePath -> CommandCleanup
|
|
cleanup file = do
|
|
-- git commit will have staged the file's content;
|
|
-- drop that and run command queued by Add.state to
|
|
-- stage the symlink
|
|
g <- Annex.gitRepo
|
|
liftIO $ Git.run g "reset" [Params "-q --", File file]
|
|
Annex.queueRun
|
|
return True
|