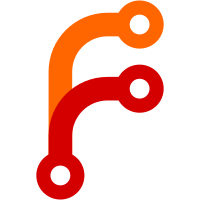
This is a first step toward that goal, using the ProposedAccepted type in RemoteConfig lets initremote/enableremote reject bad parameters that were passed in a remote's configuration, while avoiding enableremote rejecting bad parameters that have already been stored in remote.log This does not eliminate every place where a remote config is parsed and a default value is used if the parse false. But, I did fix several things that expected foo=yes/no and so confusingly accepted foo=true but treated it like foo=no. There are still some fields that are parsed with yesNo but not not checked when initializing a remote, and there are other fields that are parsed in other ways and not checked when initializing a remote. This also lays groundwork for rejecting unknown/typoed config keys.
38 lines
1.2 KiB
Haskell
38 lines
1.2 KiB
Haskell
{- git-annex assistant gpg stuff
|
|
-
|
|
- Copyright 2013 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Assistant.Gpg where
|
|
|
|
import Utility.Gpg
|
|
import Utility.UserInfo
|
|
import Types.Remote (RemoteConfigField)
|
|
import Annex.SpecialRemote.Config
|
|
import Types.ProposedAccepted
|
|
|
|
import qualified Data.Map as M
|
|
import Control.Applicative
|
|
import Prelude
|
|
|
|
{- Generates a gpg user id that is not used by any existing secret key -}
|
|
newUserId :: GpgCmd -> IO UserId
|
|
newUserId cmd = do
|
|
oldkeys <- secretKeys cmd
|
|
username <- either (const "unknown") id <$> myUserName
|
|
let basekeyname = username ++ "'s git-annex encryption key"
|
|
return $ Prelude.head $ filter (\n -> M.null $ M.filter (== n) oldkeys)
|
|
( basekeyname
|
|
: map (\n -> basekeyname ++ show n) ([2..] :: [Int])
|
|
)
|
|
|
|
data EnableEncryption = HybridEncryption | SharedEncryption | NoEncryption
|
|
deriving (Eq)
|
|
|
|
{- Generates Remote configuration for encryption. -}
|
|
configureEncryption :: EnableEncryption -> (RemoteConfigField, ProposedAccepted String)
|
|
configureEncryption SharedEncryption = (encryptionField, Proposed "shared")
|
|
configureEncryption NoEncryption = (encryptionField, Proposed "none")
|
|
configureEncryption HybridEncryption = (encryptionField, Proposed "hybrid")
|