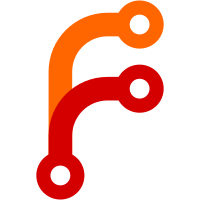
This is a git-remote-gcrypt encrypted special remote. Only sending files in to the remote works, and only for local repositories. Most of the work so far has involved making initremote work. A particular problem is that remote setup in this case needs to generate its own uuid, derivied from the gcrypt-id. That required some larger changes in the code to support. For ssh remotes, this will probably just reuse Remote.Rsync's code, so should be easy enough. And for downloading from a web remote, I will need to factor out the part of Remote.Git that does that. One particular thing that will need work is supporting hot-swapping a local gcrypt remote. I think it needs to store the gcrypt-id in the git config of the local remote, so that it can check it every time, and compare with the cached annex-uuid for the remote. If there is a mismatch, it can change both the cached annex-uuid and the gcrypt-id. That should work, and I laid some groundwork for it by already reading the remote's config when it's local. (Also needed for other reasons.) This commit was sponsored by Daniel Callahan.
98 lines
2.7 KiB
Haskell
98 lines
2.7 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2011,2013 Joey Hess <joey@kitenet.net>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.InitRemote where
|
|
|
|
import qualified Data.Map as M
|
|
|
|
import Common.Annex
|
|
import Command
|
|
import qualified Remote
|
|
import qualified Logs.Remote
|
|
import qualified Types.Remote as R
|
|
import Logs.UUID
|
|
import Logs.Trust
|
|
|
|
import Data.Ord
|
|
|
|
def :: [Command]
|
|
def = [command "initremote"
|
|
(paramPair paramName $ paramOptional $ paramRepeating paramKeyValue)
|
|
seek SectionSetup "creates a special (non-git) remote"]
|
|
|
|
seek :: [CommandSeek]
|
|
seek = [withWords start]
|
|
|
|
start :: [String] -> CommandStart
|
|
start [] = error "Specify a name for the remote."
|
|
start (name:ws) = ifM (isJust <$> findExisting name)
|
|
( error $ "There is already a special remote named \"" ++ name ++
|
|
"\". (Use enableremote to enable an existing special remote.)"
|
|
, do
|
|
let c = newConfig name
|
|
t <- findType config
|
|
|
|
showStart "initremote" name
|
|
next $ perform t name $ M.union config c
|
|
)
|
|
where
|
|
config = Logs.Remote.keyValToConfig ws
|
|
|
|
perform :: RemoteType -> String -> R.RemoteConfig -> CommandPerform
|
|
perform t name c = do
|
|
(c', u) <- R.setup t Nothing c
|
|
next $ cleanup u name c'
|
|
|
|
cleanup :: UUID -> String -> R.RemoteConfig -> CommandCleanup
|
|
cleanup u name c = do
|
|
describeUUID u name
|
|
Logs.Remote.configSet u c
|
|
return True
|
|
|
|
{- See if there's an existing special remote with this name. -}
|
|
findExisting :: String -> Annex (Maybe (UUID, R.RemoteConfig))
|
|
findExisting name = do
|
|
t <- trustMap
|
|
matches <- sortBy (comparing $ \(u, _c) -> M.lookup u t )
|
|
. findByName name
|
|
<$> Logs.Remote.readRemoteLog
|
|
return $ headMaybe matches
|
|
|
|
newConfig :: String -> R.RemoteConfig
|
|
newConfig name = M.singleton nameKey name
|
|
|
|
findByName :: String -> M.Map UUID R.RemoteConfig -> [(UUID, R.RemoteConfig)]
|
|
findByName n = filter (matching . snd) . M.toList
|
|
where
|
|
matching c = case M.lookup nameKey c of
|
|
Nothing -> False
|
|
Just n'
|
|
| n' == n -> True
|
|
| otherwise -> False
|
|
|
|
remoteNames :: Annex [String]
|
|
remoteNames = do
|
|
m <- Logs.Remote.readRemoteLog
|
|
return $ mapMaybe (M.lookup nameKey . snd) $ M.toList m
|
|
|
|
{- find the specified remote type -}
|
|
findType :: R.RemoteConfig -> Annex RemoteType
|
|
findType config = maybe unspecified specified $ M.lookup typeKey config
|
|
where
|
|
unspecified = error "Specify the type of remote with type="
|
|
specified s = case filter (findtype s) Remote.remoteTypes of
|
|
[] -> error $ "Unknown remote type " ++ s
|
|
(t:_) -> return t
|
|
findtype s i = R.typename i == s
|
|
|
|
{- The name of a configured remote is stored in its config using this key. -}
|
|
nameKey :: String
|
|
nameKey = "name"
|
|
|
|
{- The type of a remote is stored in its config using this key. -}
|
|
typeKey :: String
|
|
typeKey = "type"
|