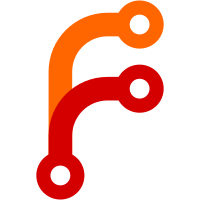
Eliminated complexity and future proofed. The most important change is that all functions over Difference are now total; any Difference that can be expressed should be handled. Avoids needs for sanity checking of inputs, and version skew with the future. Also, the difference.log now serializes a [Difference], not a Differences. This saves space and keeps it simpler. Note that [Difference] might contain conflicting differences (eg, [Version5, Version6]. In this case, one of them needs to consistently win over the others, probably based on Ord.
41 lines
1.2 KiB
Haskell
41 lines
1.2 KiB
Haskell
{- git-annex difference log
|
|
-
|
|
- Copyright 2015 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Logs.Difference (
|
|
recordDifferences,
|
|
recordedDifferences,
|
|
recordedDifferencesFor,
|
|
module Logs.Difference.Pure
|
|
) where
|
|
|
|
import Data.Monoid
|
|
import Data.Time.Clock.POSIX
|
|
import qualified Data.Map as M
|
|
|
|
import Common.Annex
|
|
import Types.Difference
|
|
import qualified Annex.Branch
|
|
import Logs
|
|
import Logs.UUIDBased
|
|
import Logs.Difference.Pure
|
|
|
|
recordDifferences :: Differences -> UUID -> Annex ()
|
|
recordDifferences (Differences differences) uuid = do
|
|
ts <- liftIO getPOSIXTime
|
|
Annex.Branch.change differenceLog $
|
|
showLog id . changeLog ts uuid (show differences) . parseLog Just
|
|
recordDifferences UnknownDifferences _ = return ()
|
|
|
|
-- Map of UUIDs that have Differences recorded.
|
|
-- If a new version of git-annex has added a Difference this version
|
|
-- doesn't know about, it will contain UnknownDifferences.
|
|
recordedDifferences :: Annex (M.Map UUID Differences)
|
|
recordedDifferences = parseDifferencesLog <$> Annex.Branch.get differenceLog
|
|
|
|
recordedDifferencesFor :: UUID -> Annex Differences
|
|
recordedDifferencesFor u = fromMaybe mempty . M.lookup u
|
|
<$> recordedDifferences
|