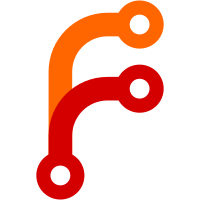
* When annex objects are received into git repositories, their checksums are verified then too. * To get the old, faster, behavior of not verifying checksums, set annex.verify=false, or remote.<name>.annex-verify=false. * setkey, rekey: These commands also now verify that the provided file matches the key, unless annex.verify=false. * reinject: Already verified content; this can now be disabled by setting annex.verify=false. recvkey and reinject already did verification, so removed now duplicate code from them. fsck still does its own verification, which is ok since it does not use getViaTmp, so verification doesn't happen twice when using fsck --from.
54 lines
1.3 KiB
Haskell
54 lines
1.3 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2011 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.Reinject where
|
|
|
|
import Common.Annex
|
|
import Command
|
|
import Logs.Location
|
|
import Annex.Content
|
|
|
|
cmd :: Command
|
|
cmd = command "reinject" SectionUtility
|
|
"sets content of annexed file"
|
|
(paramPair "SRC" "DEST") (withParams seek)
|
|
|
|
seek :: CmdParams -> CommandSeek
|
|
seek = withWords start
|
|
|
|
start :: [FilePath] -> CommandStart
|
|
start (src:dest:[])
|
|
| src == dest = stop
|
|
| otherwise =
|
|
ifAnnexed src
|
|
(error $ "cannot used annexed file as src: " ++ src)
|
|
go
|
|
where
|
|
go = do
|
|
showStart "reinject" dest
|
|
next $ whenAnnexed (perform src) dest
|
|
start _ = error "specify a src file and a dest file"
|
|
|
|
perform :: FilePath -> FilePath -> Key -> CommandPerform
|
|
perform src _dest key = ifM move
|
|
( next $ cleanup key
|
|
, error "failed"
|
|
)
|
|
where
|
|
-- The file might be on a different filesystem,
|
|
-- so moveFile is used rather than simply calling
|
|
-- moveToObjectDir; disk space is also checked this way,
|
|
-- and the file's content is verified to match the key.
|
|
move = getViaTmp DefaultVerify key $ \tmp ->
|
|
liftIO $ catchBoolIO $ do
|
|
moveFile src tmp
|
|
return True
|
|
|
|
cleanup :: Key -> CommandCleanup
|
|
cleanup key = do
|
|
logStatus key InfoPresent
|
|
return True
|