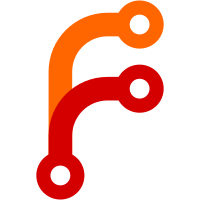
No behavior changes (hopefully), just adding SeekInput and plumbing it through to the JSON display code for later use. Over the course of 2 grueling days. withFilesNotInGit reimplemented in terms of seekHelper should be the only possible behavior change. It seems to test as behaving the same. Note that seekHelper dummies up the SeekInput in the case where segmentPaths' gives up on sorting the expanded paths because there are too many input paths. When SeekInput later gets exposed as a json field, that will result in it being a little bit wrong in the case where 100 or more paths are passed to a git-annex command. I think this is a subtle enough problem to not matter. If it does turn out to be a problem, fixing it would require splitting up the input parameters into groups of < 100, which would make git ls-files run perhaps more than is necessary. May want to revisit this, because that fix seems fairly low-impact.
76 lines
2.2 KiB
Haskell
76 lines
2.2 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2014 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.Undo where
|
|
|
|
import Command
|
|
import Git.DiffTree
|
|
import Git.FilePath
|
|
import Git.UpdateIndex
|
|
import Git.Sha
|
|
import qualified Git.LsFiles as LsFiles
|
|
import qualified Git.Command as Git
|
|
import qualified Git.Branch
|
|
import qualified Command.Sync
|
|
|
|
cmd :: Command
|
|
cmd = notBareRepo $
|
|
command "undo" SectionCommon
|
|
"undo last change to a file or directory"
|
|
paramPaths (withParams seek)
|
|
|
|
seek :: CmdParams -> CommandSeek
|
|
seek ps = do
|
|
-- Safety first; avoid any undo that would touch files that are not
|
|
-- in the index.
|
|
(fs, cleanup) <- inRepo $ LsFiles.notInRepo [] False (map toRawFilePath ps)
|
|
unless (null fs) $
|
|
giveup $ "Cannot undo changes to files that are not checked into git: " ++ unwords (map fromRawFilePath fs)
|
|
void $ liftIO $ cleanup
|
|
|
|
-- Committing staged changes before undo allows later
|
|
-- undoing the undo. It would be nicer to only commit staged
|
|
-- changes to the specified files, rather than all staged changes.
|
|
void $ Command.Sync.commitStaged Git.Branch.ManualCommit
|
|
"commit before undo"
|
|
|
|
withStrings (commandAction . start) ps
|
|
|
|
start :: FilePath -> CommandStart
|
|
start p = starting "undo" ai si $
|
|
perform p
|
|
where
|
|
ai = ActionItemOther (Just p)
|
|
si = SeekInput [p]
|
|
|
|
perform :: FilePath -> CommandPerform
|
|
perform p = do
|
|
g <- gitRepo
|
|
|
|
-- Get the reversed diff that needs to be applied to undo.
|
|
(diff, cleanup) <- inRepo $
|
|
diffLog [Param "-R", Param "--", Param p]
|
|
top <- inRepo $ toTopFilePath $ toRawFilePath p
|
|
let diff' = filter (`isDiffOf` top) diff
|
|
liftIO $ streamUpdateIndex g (map stageDiffTreeItem diff')
|
|
|
|
-- Take two passes through the diff, first doing any removals,
|
|
-- and then any adds. This order is necessary to handle eg, removing
|
|
-- a directory and replacing it with a file.
|
|
let (removals, adds) = partition (\di -> dstsha di `elem` nullShas) diff'
|
|
let mkrel di = liftIO $ relPathCwdToFile $ fromRawFilePath $
|
|
fromTopFilePath (file di) g
|
|
|
|
forM_ removals $ \di -> do
|
|
f <- mkrel di
|
|
liftIO $ nukeFile f
|
|
|
|
forM_ adds $ \di -> do
|
|
f <- mkrel di
|
|
inRepo $ Git.run [Param "checkout", Param "--", File f]
|
|
|
|
next $ liftIO cleanup
|