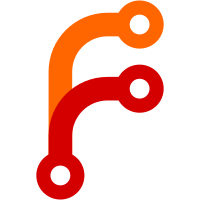
It's important that it be clear that it overrides a config, such that reloading the git config won't change it, and in particular, setConfig won't change it. Most of the calls to changeGitConfig were actually after setConfig, which was redundant and unncessary. So removed those. The only remaining one, besides --debug, is in the handling of repository-global config values. That one's ok, because the way mergeGitConfig is implemented, it does not override any value that is set in git config. If a value with a repo-global setting was passed to setConfig, it would set it in the git config, reload the git config, re-apply mergeGitConfig, and use the newly set value, which is the right thing.
63 lines
1.6 KiB
Haskell
63 lines
1.6 KiB
Haskell
{- common command-line options
|
|
-
|
|
- Copyright 2010-2011 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module CmdLine.Option where
|
|
|
|
import Options.Applicative
|
|
|
|
import Annex.Common
|
|
import CmdLine.Usage
|
|
import CmdLine.GlobalSetter
|
|
import qualified Annex
|
|
import Types.Messages
|
|
import Types.DeferredParse
|
|
|
|
-- Global options accepted by both git-annex and git-annex-shell sub-commands.
|
|
commonGlobalOptions :: [GlobalOption]
|
|
commonGlobalOptions =
|
|
[ globalFlag (setforce True)
|
|
( long "force"
|
|
<> help "allow actions that may lose annexed data"
|
|
<> hidden
|
|
)
|
|
, globalFlag (setfast True)
|
|
( long "fast" <> short 'F'
|
|
<> help "avoid slow operations"
|
|
<> hidden
|
|
)
|
|
, globalFlag (Annex.setOutput QuietOutput)
|
|
( long "quiet" <> short 'q'
|
|
<> help "avoid verbose output"
|
|
<> hidden
|
|
)
|
|
, globalFlag (Annex.setOutput NormalOutput)
|
|
( long "verbose" <> short 'v'
|
|
<> help "allow verbose output (default)"
|
|
<> hidden
|
|
)
|
|
, globalFlag setdebug
|
|
( long "debug" <> short 'd'
|
|
<> help "show debug messages"
|
|
<> hidden
|
|
)
|
|
, globalFlag unsetdebug
|
|
( long "no-debug"
|
|
<> help "don't show debug messages"
|
|
<> hidden
|
|
)
|
|
, globalSetter setforcebackend $ strOption
|
|
( long "backend" <> short 'b' <> metavar paramName
|
|
<> help "specify key-value backend to use"
|
|
<> hidden
|
|
)
|
|
]
|
|
where
|
|
setforce v = Annex.changeState $ \s -> s { Annex.force = v }
|
|
setfast v = Annex.changeState $ \s -> s { Annex.fast = v }
|
|
setforcebackend v = Annex.changeState $ \s -> s { Annex.forcebackend = Just v }
|
|
setdebug = Annex.overrideGitConfig $ \c -> c { annexDebug = True }
|
|
unsetdebug = Annex.overrideGitConfig $ \c -> c { annexDebug = False }
|