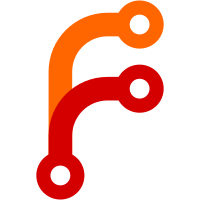
retrieveExport is part of ongoing transition to make remote methods throw exceptions, rather than silently hide them. getKey very rarely fails, and when it does it's always for the same reason (user configured annex.backend to url for some reason). So, this will avoid dealing with Nothing everywhere it's used. This commit was sponsored by Ilya Shlyakhter on Patreon.
63 lines
1.8 KiB
Haskell
63 lines
1.8 KiB
Haskell
{- git-annex "WORM" backend -- Write Once, Read Many
|
|
-
|
|
- Copyright 2010 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Backend.WORM (backends) where
|
|
|
|
import Annex.Common
|
|
import Types.Key
|
|
import Types.Backend
|
|
import Types.KeySource
|
|
import Backend.Utilities
|
|
import Git.FilePath
|
|
import Utility.Metered
|
|
|
|
import qualified Data.ByteString.Char8 as S8
|
|
import qualified Utility.RawFilePath as R
|
|
|
|
backends :: [Backend]
|
|
backends = [backend]
|
|
|
|
backend :: Backend
|
|
backend = Backend
|
|
{ backendVariety = WORMKey
|
|
, getKey = Just keyValue
|
|
, verifyKeyContent = Nothing
|
|
, canUpgradeKey = Just needsUpgrade
|
|
, fastMigrate = Just removeSpaces
|
|
, isStableKey = const True
|
|
}
|
|
|
|
{- The key includes the file size, modification time, and the
|
|
- original filename relative to the top of the git repository.
|
|
-}
|
|
keyValue :: KeySource -> MeterUpdate -> Annex Key
|
|
keyValue source _ = do
|
|
let f = contentLocation source
|
|
stat <- liftIO $ R.getFileStatus f
|
|
sz <- liftIO $ getFileSize' (fromRawFilePath f) stat
|
|
relf <- fromRawFilePath . getTopFilePath
|
|
<$> inRepo (toTopFilePath $ keyFilename source)
|
|
return $ mkKey $ \k -> k
|
|
{ keyName = genKeyName relf
|
|
, keyVariety = WORMKey
|
|
, keySize = Just sz
|
|
, keyMtime = Just $ modificationTime stat
|
|
}
|
|
|
|
{- Old WORM keys could contain spaces, and can be upgraded to remove them. -}
|
|
needsUpgrade :: Key -> Bool
|
|
needsUpgrade key = ' ' `S8.elem` fromKey keyName key
|
|
|
|
removeSpaces :: Key -> Backend -> AssociatedFile -> Annex (Maybe Key)
|
|
removeSpaces oldkey newbackend _
|
|
| migratable = return $ Just $ alterKey oldkey $ \d -> d
|
|
{ keyName = encodeBS $ reSanitizeKeyName $ decodeBS $ keyName d }
|
|
| otherwise = return Nothing
|
|
where
|
|
migratable = oldvariety == newvariety
|
|
oldvariety = fromKey keyVariety oldkey
|
|
newvariety = backendVariety newbackend
|