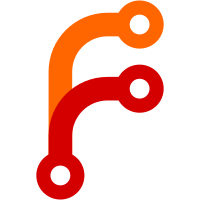
registerurl: When an url is claimed by a special remote other than the web,
update location tracking for that special remote.
registerurl's behavior was changed in commit
451171b7c1
, apparently accidentially to not
update location tracking except for the web.
This makes registerurl followed by unregisterurl not be a no-op, when the
url happens to be claimed by a remote other than the web. It is a noop when
the url is unclaimed except by the web. I don't like the inconsistency,
and wish that registerurl and unregisterurl never updated location
tracking, which would be more in keeping with them being plumbing.
But there is the fact that it used to behave this way, and also it was
inconsistent that it updated location tracking for the web but not for
other remotes, unlike addurl. And there's an argument that the user might
not know what remote to expect to claim an url, so would be considerably in
the dark when using registerurl. (Although they have to know what content
gets downloaded, since they specify a key..)
Sponsored-By: the NIH-funded NICEMAN (ReproNim TR&D3) project
68 lines
2 KiB
Haskell
68 lines
2 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2013-2021 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.RmUrl where
|
|
|
|
import Command
|
|
import Logs.Web
|
|
import Annex.WorkTree
|
|
|
|
cmd :: Command
|
|
cmd = notBareRepo $
|
|
command "rmurl" SectionCommon
|
|
"record file is not available at url"
|
|
(paramRepeating (paramPair paramFile paramUrl))
|
|
(seek <$$> optParser)
|
|
|
|
data RmUrlOptions = RmUrlOptions
|
|
{ rmThese :: CmdParams
|
|
, batchOption :: BatchMode
|
|
}
|
|
|
|
optParser :: CmdParamsDesc -> Parser RmUrlOptions
|
|
optParser desc = RmUrlOptions
|
|
<$> cmdParams desc
|
|
<*> parseBatchOption False
|
|
|
|
seek :: RmUrlOptions -> CommandSeek
|
|
seek o = case batchOption o of
|
|
Batch fmt -> batchOnly Nothing (rmThese o) $
|
|
batchInput fmt batchParser (batchCommandAction . start)
|
|
NoBatch -> withPairs (commandAction . start) (rmThese o)
|
|
|
|
-- Split on the last space, since a FilePath can contain whitespace,
|
|
-- but a url should not.
|
|
batchParser :: String -> Annex (Either String (FilePath, URLString))
|
|
batchParser s = case separate (== ' ') (reverse s) of
|
|
(ru, rf)
|
|
| null ru || null rf -> return $ Left "Expected: \"file url\""
|
|
| otherwise -> do
|
|
let f = reverse rf
|
|
f' <- liftIO $ fromRawFilePath
|
|
<$> relPathCwdToFile (toRawFilePath f)
|
|
return $ Right (f', reverse ru)
|
|
|
|
start :: (SeekInput, (FilePath, URLString)) -> CommandStart
|
|
start (si, (file, url)) = lookupKeyStaged file' >>= \case
|
|
Nothing -> stop
|
|
Just key -> do
|
|
let ai = mkActionItem (key, AssociatedFile (Just file'))
|
|
starting "rmurl" ai si $
|
|
next $ cleanup url key
|
|
where
|
|
file' = toRawFilePath file
|
|
|
|
cleanup :: String -> Key -> CommandCleanup
|
|
cleanup url key = do
|
|
-- Remove the url, no matter what downloader.
|
|
forM_ [minBound..maxBound] $ \dl ->
|
|
setUrlMissing key (setDownloader url dl)
|
|
return True
|
|
-- Unlike addurl, this does not update location tracking
|
|
-- for remotes other than the web special remote. Doing so with
|
|
-- a remote that git-annex can drop content from would rather
|
|
-- unexpectedly leave content stranded on that remote.
|