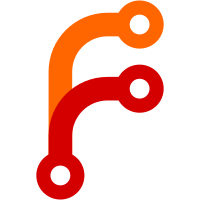
This does not change the overall license of the git-annex program, which was already AGPL due to a number of sources files being AGPL already. Legally speaking, I'm adding a new license under which these files are now available; I already released their current contents under the GPL license. Now they're dual licensed GPL and AGPL. However, I intend for all my future changes to these files to only be released under the AGPL license, and I won't be tracking the dual licensing status, so I'm simply changing the license statement to say it's AGPL. (In some cases, others wrote parts of the code of a file and released it under the GPL; but in all cases I have contributed a significant portion of the code in each file and it's that code that is getting the AGPL license; the GPL license of other contributors allows combining with AGPL code.)
55 lines
1.5 KiB
Haskell
55 lines
1.5 KiB
Haskell
{- git-annex single-value log, pure operations
|
|
-
|
|
- Copyright 2014-2019 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Logs.SingleValue.Pure where
|
|
|
|
import Annex.Common
|
|
import Logs.Line
|
|
import Annex.VectorClock
|
|
|
|
import qualified Data.Set as S
|
|
import qualified Data.ByteString as B
|
|
import qualified Data.ByteString.Lazy as L
|
|
import qualified Data.Attoparsec.ByteString.Lazy as A
|
|
import Data.Attoparsec.ByteString.Char8 (char)
|
|
import Data.ByteString.Builder
|
|
|
|
class SingleValueSerializable v where
|
|
serialize :: v -> B.ByteString
|
|
deserialize :: B.ByteString -> Maybe v
|
|
|
|
data LogEntry v = LogEntry
|
|
{ changed :: VectorClock
|
|
, value :: v
|
|
} deriving (Eq, Ord)
|
|
|
|
type Log v = S.Set (LogEntry v)
|
|
|
|
buildLog :: (SingleValueSerializable v) => Log v -> Builder
|
|
buildLog = mconcat . map genline . S.toList
|
|
where
|
|
genline (LogEntry c v) =
|
|
buildVectorClock c <> sp <> byteString (serialize v) <> nl
|
|
sp = charUtf8 ' '
|
|
nl = charUtf8 '\n'
|
|
|
|
parseLog :: (Ord v, SingleValueSerializable v) => L.ByteString -> Log v
|
|
parseLog = S.fromList . fromMaybe []
|
|
. A.maybeResult . A.parse (logParser <* A.endOfInput)
|
|
|
|
logParser :: SingleValueSerializable v => A.Parser [LogEntry v]
|
|
logParser = parseLogLines $ LogEntry
|
|
<$> vectorClockParser
|
|
<* char ' '
|
|
<*> (parsevalue =<< A.takeByteString)
|
|
where
|
|
parsevalue = maybe (fail "log line parse failure") return . deserialize
|
|
|
|
newestValue :: Log v -> Maybe v
|
|
newestValue s
|
|
| S.null s = Nothing
|
|
| otherwise = Just (value $ S.findMax s)
|