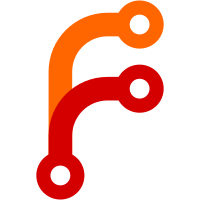
Avoid a delay at startup when concurrency is enabled and there are rsync or gcrypt special remotes, which was caused by git-annex opening a ssh connection to the remote too early. sshOptions makes a connection to the ssh server if one is not already open, when concurrency is enabled. Avoid doing that at startup, when the remote list is being built, but the remote may not be used at all. Instead, rsync/gcrypt now runs sshOptions once per ssh connection to the server. This should not be significant overhead since Remote.Git already has the same overhead (as do Bup and Ddar).
51 lines
1.1 KiB
Haskell
51 lines
1.1 KiB
Haskell
{- Rsync urls.
|
|
-
|
|
- Copyright 2014-2018 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
{-# LANGUAGE CPP #-}
|
|
|
|
module Remote.Rsync.RsyncUrl where
|
|
|
|
import Types
|
|
import Annex.Locations
|
|
import Utility.Rsync
|
|
import Utility.SafeCommand
|
|
|
|
import Data.Default
|
|
import System.FilePath.Posix
|
|
#ifdef mingw32_HOST_OS
|
|
import Utility.Split
|
|
#endif
|
|
import Annex.DirHashes
|
|
|
|
type RsyncUrl = String
|
|
|
|
data RsyncOpts = RsyncOpts
|
|
{ rsyncUrl :: RsyncUrl
|
|
, rsyncOptions :: Annex [CommandParam]
|
|
, rsyncUploadOptions :: Annex [CommandParam]
|
|
, rsyncDownloadOptions :: Annex [CommandParam]
|
|
, rsyncShellEscape :: Bool
|
|
}
|
|
|
|
rsyncEscape :: RsyncOpts -> RsyncUrl -> RsyncUrl
|
|
rsyncEscape o u
|
|
| rsyncShellEscape o && rsyncUrlIsShell (rsyncUrl o) = shellEscape u
|
|
| otherwise = u
|
|
|
|
mkRsyncUrl :: RsyncOpts -> FilePath -> RsyncUrl
|
|
mkRsyncUrl o f = rsyncUrl o </> rsyncEscape o f
|
|
|
|
rsyncUrls :: RsyncOpts -> Key -> [RsyncUrl]
|
|
rsyncUrls o k = map use dirHashes
|
|
where
|
|
use h = rsyncUrl o </> hash h </> rsyncEscape o (f </> f)
|
|
f = keyFile k
|
|
#ifndef mingw32_HOST_OS
|
|
hash h = h def k
|
|
#else
|
|
hash h = replace "\\" "/" (h def k)
|
|
#endif
|