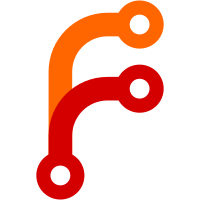
So these special remotes are always supported. IIRC these build flags were added because the dep chains were a bit too long, or perhaps because the libraries were not available in Debian stable, or something like that. That was long ago, those reasons no longer apply, and users get confused when builtin special remotes are not available, so it seems best to remove the build flags now. If this does cause a problem it can be reverted of course.. This commit was sponsored by Jochen Bartl on Patreon.
89 lines
1.8 KiB
Haskell
89 lines
1.8 KiB
Haskell
{- git-annex build flags
|
|
-
|
|
- Copyright 2013-2017 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
{-# LANGUAGE CPP #-}
|
|
|
|
module BuildFlags where
|
|
|
|
import Data.List
|
|
import Data.Ord
|
|
import qualified Data.CaseInsensitive as CI
|
|
|
|
buildFlags :: [String]
|
|
buildFlags = filter (not . null)
|
|
[ ""
|
|
#ifdef WITH_ASSISTANT
|
|
, "Assistant"
|
|
#else
|
|
#warning Building without the assistant.
|
|
#endif
|
|
#ifdef WITH_WEBAPP
|
|
, "Webapp"
|
|
#else
|
|
#warning Building without the webapp. You probably need to install Yesod..
|
|
#endif
|
|
#ifdef WITH_PAIRING
|
|
, "Pairing"
|
|
#else
|
|
#warning Building without local pairing.
|
|
#endif
|
|
#ifdef WITH_INOTIFY
|
|
, "Inotify"
|
|
#endif
|
|
#ifdef WITH_FSEVENTS
|
|
, "FsEvents"
|
|
#endif
|
|
#ifdef WITH_KQUEUE
|
|
, "Kqueue"
|
|
#endif
|
|
#ifdef WITH_DBUS
|
|
, "DBus"
|
|
#endif
|
|
#ifdef WITH_DESKTOP_NOTIFY
|
|
, "DesktopNotify"
|
|
#endif
|
|
#ifdef WITH_TORRENTPARSER
|
|
, "TorrentParser"
|
|
#endif
|
|
#ifdef WITH_MAGICMIME
|
|
, "MagicMime"
|
|
#endif
|
|
#ifdef DEBUGLOCKS
|
|
, "DebugLocks"
|
|
#endif
|
|
-- Always enabled now, but users may be used to seeing these flags
|
|
-- listed.
|
|
, "Feeds"
|
|
, "Testsuite"
|
|
, "S3"
|
|
, "WebDAV"
|
|
]
|
|
|
|
-- Not a complete list, let alone a listing transitive deps, but only
|
|
-- the ones that are often interesting to know.
|
|
dependencyVersions :: [String]
|
|
dependencyVersions = map fmt $ sortBy (comparing (CI.mk . fst))
|
|
[ ("feed", VERSION_feed)
|
|
, ("uuid", VERSION_uuid)
|
|
, ("bloomfilter", VERSION_bloomfilter)
|
|
, ("http-client", VERSION_http_client)
|
|
, ("persistent-sqlite", VERSION_persistent_sqlite)
|
|
, ("cryptonite", VERSION_cryptonite)
|
|
, ("aws", VERSION_aws)
|
|
, ("DAV", VERSION_DAV)
|
|
#ifdef WITH_TORRENTPARSER
|
|
, ("torrent", VERSION_torrent)
|
|
#endif
|
|
#ifdef WITH_WEBAPP
|
|
, ("yesod", VERSION_yesod)
|
|
#endif
|
|
#ifdef TOOL_VERSION_ghc
|
|
, ("ghc", TOOL_VERSION_ghc)
|
|
#endif
|
|
]
|
|
where
|
|
fmt (p, v) = p ++ "-" ++ v
|