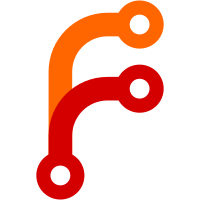
* chore: initial prototype of net api from utility process * chore: update url loader to work on both browser and utility processes * chore: add net files to utility process bundle * chore: re-add app ready check but only on main process * chore: replace browser thread dcheck's with sequence checker * refactor: move url loader from browser to common * refactor: move net-client-request.ts from browser to common * docs: add utility process to net api docs * refactor: move net module app ready check to browser only * refactor: switch import from main to common after moving to common * test: add basic net module test for utility process * refactor: switch browser pid with utility pid * refactor: move electron_api_net from browser to common * chore: add fetch to utility net module * chore: add isOnline and online to utility net module * refactor: move net spec helpers into helper file * refactor: break apart net module tests Adds two additional net module test files: `api-net-session-spec.ts` for tests that depend on a session being available (aka depend on running on the main process) and `api-net-custom-protocols-spec.ts` for custom protocol tests. This enables running `api-net-spec.ts` in the utility process. * test: add utility process mocha runner to run net module tests * docs: add utility process to net module classes * refactor: update imports in lib/utility to use electron/utility * chore: check browser context before using in main process Since the browser context supplied to the SimpleURLLoaderWrapper can now be null for use in the UtilityProcess, adding a null check for the main process before use to get a more sensible error if something goes wrong. Co-authored-by: Cheng Zhao <github@zcbenz.com> * chore: remove test debugging * chore: remove unnecessary header include * docs: add utility process net module limitations * test: run net module tests in utility process individually * refactor: clean up prior utility process net tests * chore: add resolveHost to utility process net module * chore: replace resolve host dcheck with sequence checker * test: add net module tests for net.resolveHost * docs: remove utility process limitation for resolveHost --------- Co-authored-by: deepak1556 <hop2deep@gmail.com> Co-authored-by: Cheng Zhao <github@zcbenz.com>
82 lines
2.4 KiB
C++
82 lines
2.4 KiB
C++
// Copyright (c) 2022 Microsoft, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#ifndef ELECTRON_SHELL_SERVICES_NODE_NODE_SERVICE_H_
|
|
#define ELECTRON_SHELL_SERVICES_NODE_NODE_SERVICE_H_
|
|
|
|
#include <memory>
|
|
|
|
#include "mojo/public/cpp/bindings/pending_receiver.h"
|
|
#include "mojo/public/cpp/bindings/pending_remote.h"
|
|
#include "mojo/public/cpp/bindings/receiver.h"
|
|
#include "mojo/public/cpp/bindings/remote.h"
|
|
#include "services/network/public/cpp/shared_url_loader_factory.h"
|
|
#include "services/network/public/mojom/host_resolver.mojom.h"
|
|
#include "services/network/public/mojom/url_loader_factory.mojom-forward.h"
|
|
#include "shell/services/node/public/mojom/node_service.mojom.h"
|
|
|
|
namespace node {
|
|
|
|
class Environment;
|
|
|
|
} // namespace node
|
|
|
|
namespace electron {
|
|
|
|
class ElectronBindings;
|
|
class JavascriptEnvironment;
|
|
class NodeBindings;
|
|
|
|
class URLLoaderBundle {
|
|
public:
|
|
URLLoaderBundle();
|
|
~URLLoaderBundle();
|
|
|
|
URLLoaderBundle(const URLLoaderBundle&) = delete;
|
|
URLLoaderBundle& operator=(const URLLoaderBundle&) = delete;
|
|
|
|
static URLLoaderBundle* GetInstance();
|
|
void SetURLLoaderFactory(
|
|
mojo::PendingRemote<network::mojom::URLLoaderFactory> factory,
|
|
mojo::Remote<network::mojom::HostResolver> host_resolver);
|
|
scoped_refptr<network::SharedURLLoaderFactory> GetSharedURLLoaderFactory();
|
|
network::mojom::HostResolver* GetHostResolver();
|
|
|
|
private:
|
|
scoped_refptr<network::SharedURLLoaderFactory> factory_;
|
|
mojo::Remote<network::mojom::HostResolver> host_resolver_;
|
|
};
|
|
|
|
class NodeService : public node::mojom::NodeService {
|
|
public:
|
|
explicit NodeService(
|
|
mojo::PendingReceiver<node::mojom::NodeService> receiver);
|
|
~NodeService() override;
|
|
|
|
NodeService(const NodeService&) = delete;
|
|
NodeService& operator=(const NodeService&) = delete;
|
|
|
|
// mojom::NodeService implementation:
|
|
void Initialize(node::mojom::NodeServiceParamsPtr params) override;
|
|
|
|
private:
|
|
bool node_env_stopped_ = false;
|
|
|
|
const std::unique_ptr<NodeBindings> node_bindings_;
|
|
|
|
// depends-on: node_bindings_'s uv_loop
|
|
const std::unique_ptr<ElectronBindings> electron_bindings_;
|
|
|
|
// depends-on: node_bindings_'s uv_loop
|
|
std::unique_ptr<JavascriptEnvironment> js_env_;
|
|
|
|
// depends-on: js_env_'s isolate
|
|
std::shared_ptr<node::Environment> node_env_;
|
|
|
|
mojo::Receiver<node::mojom::NodeService> receiver_{this};
|
|
};
|
|
|
|
} // namespace electron
|
|
|
|
#endif // ELECTRON_SHELL_SERVICES_NODE_NODE_SERVICE_H_
|