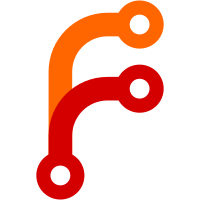
Previously electron-prebuilt would download the prebuilt binaries for the architecture native to the platform. However there are cases where being able to download for another architecture is favourable. This patch adds support for setting the architecture through npm's --arch= parameter. (Issue #53)
41 lines
1.2 KiB
JavaScript
Executable file
41 lines
1.2 KiB
JavaScript
Executable file
#!/usr/bin/env node
|
|
|
|
// maintainer note - x.y.z-ab version in package.json -> x.y.z
|
|
var version = require('./package').version.replace(/-.*/, '')
|
|
|
|
var fs = require('fs')
|
|
var os = require('os')
|
|
var path = require('path')
|
|
var extract = require('extract-zip')
|
|
var download = require('electron-download')
|
|
|
|
var platform = os.platform()
|
|
|
|
function onerror (err) {
|
|
throw err
|
|
}
|
|
|
|
var paths = {
|
|
darwin: path.join(__dirname, './dist/Electron.app/Contents/MacOS/Electron'),
|
|
linux: path.join(__dirname, './dist/electron'),
|
|
win32: path.join(__dirname, './dist/electron.exe')
|
|
}
|
|
|
|
if (!paths[platform]) throw new Error('Unknown platform: ' + platform)
|
|
|
|
if (process.env.npm_config_arch) {
|
|
download({version: version, arch: process.env.npm_config_arch}, extractFile)
|
|
} else {
|
|
download({version: version}, extractFile)
|
|
}
|
|
|
|
// unzips and makes path.txt point at the correct executable
|
|
function extractFile (err, zipPath) {
|
|
if (err) return onerror(err)
|
|
fs.writeFile(path.join(__dirname, 'path.txt'), paths[platform], function (err) {
|
|
if (err) return onerror(err)
|
|
extract(zipPath, {dir: path.join(__dirname, 'dist')}, function (err) {
|
|
if (err) return onerror(err)
|
|
})
|
|
})
|
|
}
|