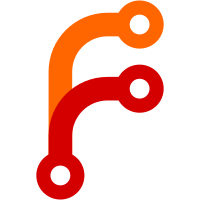
* refactor: use mojo for electron internal IPC * add sender_id, drop MessageSync * remove usages of AtomFrameMsg_Message * iwyu * first draft of renderer->browser direction * refactor to reuse a single ipc interface * implement TakeHeapSnapshot through mojo * the rest of the owl^WtakeHeapSnapshot mojofication * remove no-op overrides in AtomRendererClient * delete renderer-side ElectronApiServiceImpl when its pipe is destroyed * looks like we don't need to overlay the renderer manifest after all * don't try to send 2 replies to a sync rpc * undo changes to manifests.cc * unify sandboxed + unsandboxed ipc events * lint * register ElectronBrowser mojo service on devtools WebContents * fix takeHeapSnapshopt failure paths * {electron_api => atom}::mojom * add send_to_all to ElectronRenderer::Message * keep interface alive until callback is called * review comments * use GetContext from RendererClientBase * robustify a test that uses window.open * MessageSync posts a task to put sync messages in the same queue as async ones * add v8::MicrotasksScope and node::CallbackScope * iwyu * use weakptr to api::WebContents instead of Unretained * make MessageSync an asynchronous message & use non-associated interface * iwyu + comments * remove unused WeakPtrFactory * inline OnRendererMessage[Sync] * cleanups & comments * use helper methods instead of inline lambdas * remove unneeded async in test * add mojo to manifests deps * add gn check for //electron/manifests and mojo * don't register renderer side service until preload has been run * update gn check targets list * move interface registration back to RenderFrameCreated
49 lines
1.8 KiB
C++
49 lines
1.8 KiB
C++
// Copyright (c) 2019 GitHub, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#include "atom/app/manifests.h"
|
|
|
|
#include "base/no_destructor.h"
|
|
#include "electron/atom/common/api/api.mojom.h"
|
|
#include "printing/buildflags/buildflags.h"
|
|
#include "services/proxy_resolver/public/cpp/manifest.h"
|
|
#include "services/service_manager/public/cpp/manifest_builder.h"
|
|
|
|
#if BUILDFLAG(ENABLE_PRINTING)
|
|
#include "components/services/pdf_compositor/public/cpp/manifest.h"
|
|
#endif
|
|
|
|
#if BUILDFLAG(ENABLE_PRINT_PREVIEW)
|
|
#include "chrome/services/printing/public/cpp/manifest.h"
|
|
#endif
|
|
|
|
const service_manager::Manifest& GetElectronContentBrowserOverlayManifest() {
|
|
static base::NoDestructor<service_manager::Manifest> manifest{
|
|
service_manager::ManifestBuilder()
|
|
.WithDisplayName("Electron (browser process)")
|
|
.RequireCapability("device", "device:geolocation_control")
|
|
.RequireCapability("proxy_resolver", "factory")
|
|
.RequireCapability("chrome_printing", "converter")
|
|
.RequireCapability("pdf_compositor", "compositor")
|
|
.ExposeInterfaceFilterCapability_Deprecated(
|
|
"navigation:frame", "renderer",
|
|
service_manager::Manifest::InterfaceList<
|
|
atom::mojom::ElectronBrowser>())
|
|
.Build()};
|
|
return *manifest;
|
|
}
|
|
|
|
const std::vector<service_manager::Manifest>&
|
|
GetElectronPackagedServicesOverlayManifest() {
|
|
static base::NoDestructor<std::vector<service_manager::Manifest>> manifests{{
|
|
proxy_resolver::GetManifest(),
|
|
#if BUILDFLAG(ENABLE_PRINTING)
|
|
printing::GetPdfCompositorManifest(),
|
|
#endif
|
|
#if BUILDFLAG(ENABLE_PRINT_PREVIEW)
|
|
GetChromePrintingManifest(),
|
|
#endif
|
|
}};
|
|
return *manifests;
|
|
}
|