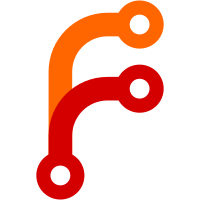
* Pipe data into HTTP protocol handlers * Remove unused parameters * Remove "sending request of http protocol urls" test Sending request to "http://" in "file://" violates CORS rules and always fail, before NetworkService somehow Chromium still sent a request even though the request failed with CORS error, so the test passes while the test is not valid. With NetworkService no request is sent at all and the test jsut fails. So this is an ancient invalid test, as sending http requests have been fully covered in other tests, I am removing this test.
80 lines
2.9 KiB
C++
80 lines
2.9 KiB
C++
// Copyright (c) 2019 GitHub, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#ifndef ATOM_BROWSER_NET_URL_PIPE_LOADER_H_
|
|
#define ATOM_BROWSER_NET_URL_PIPE_LOADER_H_
|
|
|
|
#include <memory>
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#include "mojo/public/cpp/bindings/strong_binding.h"
|
|
#include "mojo/public/cpp/system/string_data_pipe_producer.h"
|
|
#include "services/network/public/cpp/simple_url_loader.h"
|
|
#include "services/network/public/cpp/simple_url_loader_stream_consumer.h"
|
|
#include "services/network/public/mojom/url_loader.mojom.h"
|
|
|
|
namespace network {
|
|
class SharedURLLoaderFactory;
|
|
}
|
|
|
|
namespace atom {
|
|
|
|
// Read data from URL and pipe it to NetworkService.
|
|
//
|
|
// Different from creating a new loader for the URL directly, protocol handlers
|
|
// using this loader can work around CORS restrictions.
|
|
//
|
|
// This class manages its own lifetime and should delete itself when the
|
|
// connection is lost or finished.
|
|
class URLPipeLoader : public network::mojom::URLLoader,
|
|
public network::SimpleURLLoaderStreamConsumer {
|
|
public:
|
|
URLPipeLoader(scoped_refptr<network::SharedURLLoaderFactory> factory,
|
|
std::unique_ptr<network::ResourceRequest> request,
|
|
network::mojom::URLLoaderRequest loader,
|
|
network::mojom::URLLoaderClientPtr client,
|
|
const net::NetworkTrafficAnnotationTag& annotation);
|
|
|
|
private:
|
|
~URLPipeLoader() override;
|
|
|
|
void Start(scoped_refptr<network::SharedURLLoaderFactory> factory,
|
|
std::unique_ptr<network::ResourceRequest> request,
|
|
const net::NetworkTrafficAnnotationTag& annotation);
|
|
void NotifyComplete(int result);
|
|
void OnResponseStarted(const GURL& final_url,
|
|
const network::ResourceResponseHead& response_head);
|
|
void OnWrite(base::OnceClosure resume, MojoResult result);
|
|
|
|
// SimpleURLLoaderStreamConsumer:
|
|
void OnDataReceived(base::StringPiece string_piece,
|
|
base::OnceClosure resume) override;
|
|
void OnComplete(bool success) override;
|
|
void OnRetry(base::OnceClosure start_retry) override;
|
|
|
|
// URLLoader:
|
|
void FollowRedirect(const std::vector<std::string>& removed_headers,
|
|
const net::HttpRequestHeaders& modified_headers,
|
|
const base::Optional<GURL>& new_url) override {}
|
|
void ProceedWithResponse() override {}
|
|
void SetPriority(net::RequestPriority priority,
|
|
int32_t intra_priority_value) override {}
|
|
void PauseReadingBodyFromNet() override {}
|
|
void ResumeReadingBodyFromNet() override {}
|
|
|
|
mojo::Binding<network::mojom::URLLoader> binding_;
|
|
network::mojom::URLLoaderClientPtr client_;
|
|
|
|
std::unique_ptr<mojo::StringDataPipeProducer> producer_;
|
|
std::unique_ptr<network::SimpleURLLoader> loader_;
|
|
|
|
base::WeakPtrFactory<URLPipeLoader> weak_factory_;
|
|
|
|
DISALLOW_COPY_AND_ASSIGN(URLPipeLoader);
|
|
};
|
|
|
|
} // namespace atom
|
|
|
|
#endif // ATOM_BROWSER_NET_URL_PIPE_LOADER_H_
|