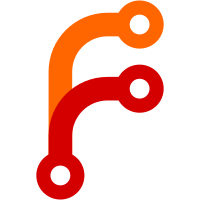
* fix: use render client id to track deleted render process hosts Instead of relying on OS process id, which may not be unique when a process is reused, we rely on the renderer client id passed by the content layer when starting the renderer process which is guaranteed to be unique for the lifetime of the app. * fix: store context id as int64_t Ensuring that it doesn't wrap easily with a large number of context creation on some malformed web pages.
32 lines
899 B
HTML
32 lines
899 B
HTML
<!DOCTYPE html>
|
|
<html>
|
|
<head>
|
|
<meta charset="utf-8">
|
|
<title></title>
|
|
<script>
|
|
const {ipcRenderer, remote} = require('electron')
|
|
|
|
const contents = remote.getCurrentWebContents()
|
|
|
|
// This should not trigger a dereference and a remote getURL call should not fail
|
|
contents.emit('render-view-deleted', {}, 'not-a-process-id')
|
|
try {
|
|
contents.getURL()
|
|
} catch (error) {
|
|
ipcRenderer.send('error-message', 'Unexpected error on getURL call')
|
|
}
|
|
|
|
// This should trigger a dereference and a remote getURL call should fail
|
|
contents.emit('render-view-deleted', {}, contents.getProcessId())
|
|
try {
|
|
contents.getURL()
|
|
ipcRenderer.send('error-message', 'No error thrown')
|
|
} catch (error) {
|
|
ipcRenderer.send('error-message', error.message)
|
|
}
|
|
</script>
|
|
</head>
|
|
<body>
|
|
|
|
</body>
|
|
</html>
|