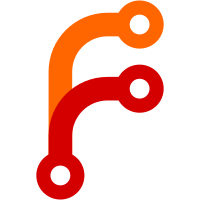
* chore: fix cpplint 'include_what_you_use' warnings Typically by including <memory>, <utility> etc. * chore: fix 'static/global string constant' warning Use C style strings instead of std::string. Style guide forbids non-trivial static / global variables. https://google.github.io/styleguide/cppguide.html#Static_and_Global_Variables /home/charles/electron/electron-gn/src/electron/script/cpplint.js * refactor: remove global string variables. Fix 'global string variables are not permitted' linter warnings by using the base::NoDestructor<> wrapper to make it explicit that these variables are never destroyed. The style guide's take on globals with nontrivial destructors: https://google.github.io/styleguide/cppguide.html#Static_and_Global_Variables * fix: initializer error introduced in last commit * fix: remove WIP file that was included by accident * fix: include order * fix: include order * fix: include order * fix: include order, again
39 lines
949 B
C++
39 lines
949 B
C++
// Copyright (c) 2017 GitHub, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#ifndef ATOM_RENDERER_WEB_WORKER_OBSERVER_H_
|
|
#define ATOM_RENDERER_WEB_WORKER_OBSERVER_H_
|
|
|
|
#include <memory>
|
|
|
|
#include "base/macros.h"
|
|
#include "v8/include/v8.h"
|
|
|
|
namespace atom {
|
|
|
|
class AtomBindings;
|
|
class NodeBindings;
|
|
|
|
// Watches for WebWorker and insert node integration to it.
|
|
class WebWorkerObserver {
|
|
public:
|
|
// Returns the WebWorkerObserver for current worker thread.
|
|
static WebWorkerObserver* GetCurrent();
|
|
|
|
void ContextCreated(v8::Local<v8::Context> context);
|
|
void ContextWillDestroy(v8::Local<v8::Context> context);
|
|
|
|
private:
|
|
WebWorkerObserver();
|
|
~WebWorkerObserver();
|
|
|
|
std::unique_ptr<NodeBindings> node_bindings_;
|
|
std::unique_ptr<AtomBindings> atom_bindings_;
|
|
|
|
DISALLOW_COPY_AND_ASSIGN(WebWorkerObserver);
|
|
};
|
|
|
|
} // namespace atom
|
|
|
|
#endif // ATOM_RENDERER_WEB_WORKER_OBSERVER_H_
|