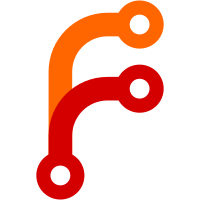
* spec: replace assert with expect in api-browser-view-spec.js * spec: replace assert with expect in api-touch-bar-spec.js * spec: replace assert with expect in api-web-frame-spec.js * spec: replace assert with expect in api-web-contents-view-spec.js * spec: replace assert with expect in security-warnings-spec.js * spec: replace assert with expect in api-menu-item-spec.js * spec: replace assert with expect in api-web-request-spec.js * spec: replace assert with expect in api-remote-spec.js * spec: replace assert with expect in api-session-spec.js * spec: replace assert with expect in api-system-preferences-spec.js * spec: replace assert with expect in api-browser-window-spec.js * spec: replace assert with expect in webview-spec.js * spec: replace assert with expect in api-net-spec.js * spec: replace assert with expect in api-protocol-spec.js * spec: replace assert with expect api-web-contents-spec.js * spec: replace assert with expect in api-shell-spec.js * spec: replace assert with expect in modules-spec.js * spec: replace assert with expect in chromium-spec.js * spec: replace assert with expect in api-crash-reporter-spec.js * spec: replace assert with expect in asar-spec.js * spec: rename assert-helpers to expect-helpers * address PR feedback
44 lines
1.4 KiB
JavaScript
44 lines
1.4 KiB
JavaScript
'use strict'
|
|
|
|
const chai = require('chai')
|
|
const ChildProcess = require('child_process')
|
|
const dirtyChai = require('dirty-chai')
|
|
const path = require('path')
|
|
const { emittedOnce } = require('./events-helpers')
|
|
const { closeWindow } = require('./window-helpers')
|
|
|
|
const { remote } = require('electron')
|
|
const { webContents, TopLevelWindow, WebContentsView } = remote
|
|
|
|
const { expect } = chai
|
|
chai.use(dirtyChai)
|
|
|
|
describe('WebContentsView', () => {
|
|
let w = null
|
|
afterEach(() => closeWindow(w).then(() => { w = null }))
|
|
|
|
it('can be used as content view', () => {
|
|
const web = webContents.create({})
|
|
w = new TopLevelWindow({ show: false })
|
|
w.setContentView(new WebContentsView(web))
|
|
})
|
|
|
|
it('prevents adding same WebContents', () => {
|
|
const web = webContents.create({})
|
|
w = new TopLevelWindow({ show: false })
|
|
w.setContentView(new WebContentsView(web))
|
|
expect(() => {
|
|
w.setContentView(new WebContentsView(web))
|
|
}).to.throw('The WebContents has already been added to a View')
|
|
})
|
|
|
|
describe('new WebContentsView()', () => {
|
|
it('does not crash on exit', async () => {
|
|
const appPath = path.join(__dirname, 'fixtures', 'api', 'leak-exit-webcontentsview.js')
|
|
const electronPath = remote.getGlobal('process').execPath
|
|
const appProcess = ChildProcess.spawn(electronPath, [appPath])
|
|
const [code] = await emittedOnce(appProcess, 'close')
|
|
expect(code).to.equal(0)
|
|
})
|
|
})
|
|
})
|