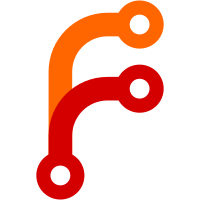
* chore: initial prototype of net api from utility process * chore: update url loader to work on both browser and utility processes * chore: add net files to utility process bundle * chore: re-add app ready check but only on main process * chore: replace browser thread dcheck's with sequence checker * refactor: move url loader from browser to common * refactor: move net-client-request.ts from browser to common * docs: add utility process to net api docs * refactor: move net module app ready check to browser only * refactor: switch import from main to common after moving to common * test: add basic net module test for utility process * refactor: switch browser pid with utility pid * refactor: move electron_api_net from browser to common * chore: add fetch to utility net module * chore: add isOnline and online to utility net module * refactor: move net spec helpers into helper file * refactor: break apart net module tests Adds two additional net module test files: `api-net-session-spec.ts` for tests that depend on a session being available (aka depend on running on the main process) and `api-net-custom-protocols-spec.ts` for custom protocol tests. This enables running `api-net-spec.ts` in the utility process. * test: add utility process mocha runner to run net module tests * docs: add utility process to net module classes * refactor: update imports in lib/utility to use electron/utility * chore: check browser context before using in main process Since the browser context supplied to the SimpleURLLoaderWrapper can now be null for use in the UtilityProcess, adding a null check for the main process before use to get a more sensible error if something goes wrong. Co-authored-by: Cheng Zhao <github@zcbenz.com> * chore: remove test debugging * chore: remove unnecessary header include * docs: add utility process net module limitations * test: run net module tests in utility process individually * refactor: clean up prior utility process net tests * chore: add resolveHost to utility process net module * chore: replace resolve host dcheck with sequence checker * test: add net module tests for net.resolveHost * docs: remove utility process limitation for resolveHost --------- Co-authored-by: deepak1556 <hop2deep@gmail.com> Co-authored-by: Cheng Zhao <github@zcbenz.com>
52 lines
1.7 KiB
TypeScript
52 lines
1.7 KiB
TypeScript
import { EventEmitter } from 'events';
|
|
import { pathToFileURL } from 'url';
|
|
|
|
import { ParentPort } from '@electron/internal/utility/parent-port';
|
|
|
|
const v8Util = process._linkedBinding('electron_common_v8_util');
|
|
|
|
const entryScript: string = v8Util.getHiddenValue(process, '_serviceStartupScript');
|
|
// We modified the original process.argv to let node.js load the init.js,
|
|
// we need to restore it here.
|
|
process.argv.splice(1, 1, entryScript);
|
|
|
|
// Clear search paths.
|
|
require('../common/reset-search-paths');
|
|
|
|
// Import common settings.
|
|
require('@electron/internal/common/init');
|
|
|
|
process._linkedBinding('electron_browser_event_emitter').setEventEmitterPrototype(EventEmitter.prototype);
|
|
|
|
const parentPort: ParentPort = new ParentPort();
|
|
Object.defineProperty(process, 'parentPort', {
|
|
enumerable: true,
|
|
writable: false,
|
|
value: parentPort
|
|
});
|
|
|
|
// Based on third_party/electron_node/lib/internal/worker/io.js
|
|
parentPort.on('newListener', (name: string) => {
|
|
if (name === 'message' && parentPort.listenerCount('message') === 0) {
|
|
parentPort.start();
|
|
}
|
|
});
|
|
|
|
parentPort.on('removeListener', (name: string) => {
|
|
if (name === 'message' && parentPort.listenerCount('message') === 0) {
|
|
parentPort.pause();
|
|
}
|
|
});
|
|
|
|
// Finally load entry script.
|
|
const { loadESM } = __non_webpack_require__('internal/process/esm_loader');
|
|
const mainEntry = pathToFileURL(entryScript);
|
|
loadESM(async (esmLoader: any) => {
|
|
try {
|
|
await esmLoader.import(mainEntry.toString(), undefined, Object.create(null));
|
|
} catch (err) {
|
|
// @ts-ignore internalBinding is a secret internal global that we shouldn't
|
|
// really be using, so we ignore the type error instead of declaring it in types
|
|
internalBinding('errors').triggerUncaughtException(err);
|
|
}
|
|
});
|