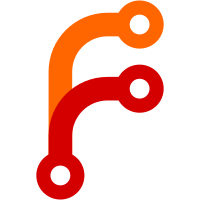
* refactor: move mate::Event to gin * refactor: move mate::Locker to gin * refactor: convert contextBridge to gin * refactor: convert contentTracing to gin * refactor: remove callback converter of native_mate * refactor: remove file_dialog_converter and native_window_converter from native_mate * refactor: convert webFrame to gin * refactor: move blink_converter to gin * refactor: remove net_converter from native_mate * refactor: remove event_emitter_caller_deprecated * refactor: remove gurl_converter from native_mate * refactor: remove file_path and string16_converter from native_mate * refactor: remove image_converter from native_mate * refactor: move value_converter to gin
77 lines
2.4 KiB
C++
77 lines
2.4 KiB
C++
// Copyright (c) 2014 GitHub, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#include "shell/common/gin_converters/value_converter.h"
|
|
|
|
#include <memory>
|
|
#include <utility>
|
|
|
|
#include "base/values.h"
|
|
#include "shell/common/v8_value_converter.h"
|
|
|
|
namespace gin {
|
|
|
|
bool Converter<base::DictionaryValue>::FromV8(v8::Isolate* isolate,
|
|
v8::Local<v8::Value> val,
|
|
base::DictionaryValue* out) {
|
|
electron::V8ValueConverter converter;
|
|
std::unique_ptr<base::Value> value(
|
|
converter.FromV8Value(val, isolate->GetCurrentContext()));
|
|
if (value && value->is_dict()) {
|
|
out->Swap(static_cast<base::DictionaryValue*>(value.get()));
|
|
return true;
|
|
} else {
|
|
return false;
|
|
}
|
|
}
|
|
|
|
v8::Local<v8::Value> Converter<base::DictionaryValue>::ToV8(
|
|
v8::Isolate* isolate,
|
|
const base::DictionaryValue& val) {
|
|
electron::V8ValueConverter converter;
|
|
return converter.ToV8Value(&val, isolate->GetCurrentContext());
|
|
}
|
|
|
|
bool Converter<base::Value>::FromV8(v8::Isolate* isolate,
|
|
v8::Local<v8::Value> val,
|
|
base::Value* out) {
|
|
electron::V8ValueConverter converter;
|
|
std::unique_ptr<base::Value> value(
|
|
converter.FromV8Value(val, isolate->GetCurrentContext()));
|
|
if (value) {
|
|
*out = std::move(*value);
|
|
return true;
|
|
} else {
|
|
return false;
|
|
}
|
|
}
|
|
|
|
v8::Local<v8::Value> Converter<base::Value>::ToV8(v8::Isolate* isolate,
|
|
const base::Value& val) {
|
|
electron::V8ValueConverter converter;
|
|
return converter.ToV8Value(&val, isolate->GetCurrentContext());
|
|
}
|
|
|
|
bool Converter<base::ListValue>::FromV8(v8::Isolate* isolate,
|
|
v8::Local<v8::Value> val,
|
|
base::ListValue* out) {
|
|
electron::V8ValueConverter converter;
|
|
std::unique_ptr<base::Value> value(
|
|
converter.FromV8Value(val, isolate->GetCurrentContext()));
|
|
if (value->is_list()) {
|
|
out->Swap(static_cast<base::ListValue*>(value.get()));
|
|
return true;
|
|
} else {
|
|
return false;
|
|
}
|
|
}
|
|
|
|
v8::Local<v8::Value> Converter<base::ListValue>::ToV8(
|
|
v8::Isolate* isolate,
|
|
const base::ListValue& val) {
|
|
electron::V8ValueConverter converter;
|
|
return converter.ToV8Value(&val, isolate->GetCurrentContext());
|
|
}
|
|
|
|
} // namespace gin
|