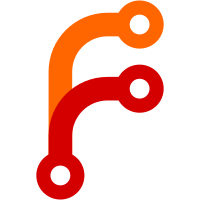
refactor: take a uint8_t span in ValidateIntegrityOrDie() Doing some groundwork for fixing unsafe base::File() APIs: - Change ValidateIntegrityOrDie() to take a span<const uint8_t> arg. We'll need this to migrate asar's base::File API calls away from the ones tagged `UNSAFE_BUFFER_USAGE` because the safe counterparts use span<uint8_t> too. - Simplify ValidateIntegrityOrDie()'s implementation by using crypto::SHA256Hash() instead of reinventing the wheel.
39 lines
1.1 KiB
C++
39 lines
1.1 KiB
C++
// Copyright (c) 2015 GitHub, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#ifndef ELECTRON_SHELL_COMMON_ASAR_ASAR_UTIL_H_
|
|
#define ELECTRON_SHELL_COMMON_ASAR_ASAR_UTIL_H_
|
|
|
|
#include <memory>
|
|
#include <string>
|
|
|
|
#include "base/containers/span.h"
|
|
|
|
namespace base {
|
|
class FilePath;
|
|
}
|
|
|
|
namespace asar {
|
|
|
|
class Archive;
|
|
struct IntegrityPayload;
|
|
|
|
// Gets or creates and caches a new Archive from the path.
|
|
std::shared_ptr<Archive> GetOrCreateAsarArchive(const base::FilePath& path);
|
|
|
|
// Separates the path to Archive out.
|
|
bool GetAsarArchivePath(const base::FilePath& full_path,
|
|
base::FilePath* asar_path,
|
|
base::FilePath* relative_path,
|
|
bool allow_root = false);
|
|
|
|
// Same with base::ReadFileToString but supports asar Archive.
|
|
bool ReadFileToString(const base::FilePath& path, std::string* contents);
|
|
|
|
void ValidateIntegrityOrDie(base::span<const uint8_t> input,
|
|
const IntegrityPayload& integrity);
|
|
|
|
} // namespace asar
|
|
|
|
#endif // ELECTRON_SHELL_COMMON_ASAR_ASAR_UTIL_H_
|