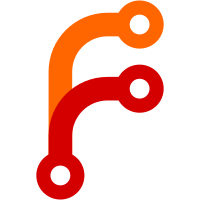
* fix: backport patch to fix systemd unit activation in Chromium This backports a patch from Chromium, which fixes systemd unit activation. That is, a globalShortcuts feature that Chromium has needs to create a systemd unit and rename it properly. Portal's global shortcuts uses that name afterwards to map the app with the shortcuts bound. However, there might be a race between Chromium binding shortcuts and renaming the unit. This is a first step to add Portal's globalShortcuts to Electron. * feat: Support global shortcuts via GlobalShortcutsPortal feature Chromium has a new feature called GlobalShortcutsPortal. It allows clients to use Portal's globalShortcuts to register and listen to shortcuts. This patches adds necessary bits, which allows Electron to use that feature. In order to make it work, one has to add --enable-features=GlobalShortcutsPortal Test: tested manually with a sample app. * docs: add GlobalShortcutsPortal feature to globalShortcuts docs Electron supports Portal's globalShortcuts API now via Chromium, and Electron apps can use that in a Wayland session. Update the docs with the required feature flag that must be passed to be able to use that implementation.
69 lines
2.3 KiB
C++
69 lines
2.3 KiB
C++
// Copyright (c) 2014 GitHub, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#ifndef ELECTRON_SHELL_BROWSER_API_ELECTRON_API_GLOBAL_SHORTCUT_H_
|
|
#define ELECTRON_SHELL_BROWSER_API_ELECTRON_API_GLOBAL_SHORTCUT_H_
|
|
|
|
#include <map>
|
|
#include <vector>
|
|
|
|
#include "base/functional/callback_forward.h"
|
|
#include "chrome/browser/extensions/global_shortcut_listener.h"
|
|
#include "extensions/common/extension_id.h"
|
|
#include "gin/wrappable.h"
|
|
#include "ui/base/accelerators/accelerator.h"
|
|
|
|
namespace gin {
|
|
template <typename T>
|
|
class Handle;
|
|
} // namespace gin
|
|
|
|
namespace electron::api {
|
|
|
|
class GlobalShortcut final
|
|
: private extensions::GlobalShortcutListener::Observer,
|
|
public gin::Wrappable<GlobalShortcut> {
|
|
public:
|
|
static gin::Handle<GlobalShortcut> Create(v8::Isolate* isolate);
|
|
|
|
// gin::Wrappable
|
|
static gin::WrapperInfo kWrapperInfo;
|
|
gin::ObjectTemplateBuilder GetObjectTemplateBuilder(
|
|
v8::Isolate* isolate) override;
|
|
const char* GetTypeName() override;
|
|
|
|
// disable copy
|
|
GlobalShortcut(const GlobalShortcut&) = delete;
|
|
GlobalShortcut& operator=(const GlobalShortcut&) = delete;
|
|
|
|
protected:
|
|
explicit GlobalShortcut(v8::Isolate* isolate);
|
|
~GlobalShortcut() override;
|
|
|
|
private:
|
|
typedef std::map<ui::Accelerator, base::RepeatingClosure>
|
|
AcceleratorCallbackMap;
|
|
typedef std::map<std::string, base::RepeatingClosure> CommandCallbackMap;
|
|
|
|
bool RegisterAll(const std::vector<ui::Accelerator>& accelerators,
|
|
const base::RepeatingClosure& callback);
|
|
bool Register(const ui::Accelerator& accelerator,
|
|
const base::RepeatingClosure& callback);
|
|
bool IsRegistered(const ui::Accelerator& accelerator);
|
|
void Unregister(const ui::Accelerator& accelerator);
|
|
void UnregisterSome(const std::vector<ui::Accelerator>& accelerators);
|
|
void UnregisterAll();
|
|
|
|
// GlobalShortcutListener::Observer implementation.
|
|
void OnKeyPressed(const ui::Accelerator& accelerator) override;
|
|
void ExecuteCommand(const extensions::ExtensionId& extension_id,
|
|
const std::string& command_id) override;
|
|
|
|
AcceleratorCallbackMap accelerator_callback_map_;
|
|
CommandCallbackMap command_callback_map_;
|
|
};
|
|
|
|
} // namespace electron::api
|
|
|
|
#endif // ELECTRON_SHELL_BROWSER_API_ELECTRON_API_GLOBAL_SHORTCUT_H_
|