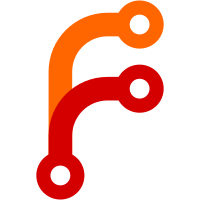
* chore: bump chromium in DEPS to 91c9f44297abe2844f593ec7956e6ce79c81f463 * chore: update chromium patches * chore: update v8 patches * build: service_names.mojom has been deleted Refs: https://chromium-review.googlesource.com/c/chromium/src/+/2568681 * chore: add DISPLAY_CAPTURE permission to converter Refs: https://chromium-review.googlesource.com/c/chromium/src/+/2551098 * chore: handle AXPropertyFilter::SCRIPT in accessibility_ui Refs: https://chromium-review.googlesource.com/c/chromium/src/+/2563923 * refactor: web_isolated_world_ids.h has been deleted Refs: https://chromium-review.googlesource.com/c/chromium/src/+/2585255 * refactor: ResourceType has been deprecated / removed in ExtensionsBrowserClient Refs: https://chromium-review.googlesource.com/c/chromium/src/+/2562002 * chore: fix lint * chore: remove deleted headers * build: disable gn check for blink header * fix: refactor X11 event handling Refs: https://chromium-review.googlesource.com/c/chromium/src/+/2577887 Refs: https://chromium-review.googlesource.com/c/chromium/src/+/2585750 * chore: update patches * chore: bump chromium in DEPS to bfd8e7dbd37af8b1bc40d887815edd5a29496fa3 * chore: update patches * refactor: xeventobserver is now x11:eventobserver Refs: https://chromium-review.googlesource.com/c/chromium/src/+/2585750 * refactor: remove UseWebUIBindingsForURL Refs: https://chromium-review.googlesource.com/c/chromium/src/+/2583590 * chore: DidProcessXEvent has been removed * chore: bump chromium in DEPS to b13e791d7244a08d9d61dbfa2bb2b6cdf1ff6294 * chore: update patches * build: change gfx::GetAtom to x11:GetAtom Refs:d972a0ae4a
* build: change gfx namespace to x11 Ref:d972a0ae4a
* build: change ui namespace to x11 Refs:c38f8571a8
:ui/gfx/x/xproto_util.h;dlc=ba9145d0c7f2b10e869e2ba482ca05b75ca35812 * chore: add patch to fix blink prefs fetching during frame swap * chore: fix lint * fix: do not make invalid SKImageRep in FrameSubscriber Refs: https://chromium-review.googlesource.com/c/chromium/src/+/2572896 Co-authored-by: Samuel Attard <samuel.r.attard@gmail.com>
90 lines
2.9 KiB
C++
90 lines
2.9 KiB
C++
// Copyright (c) 2014 GitHub, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#include "shell/browser/ui/x/x_window_utils.h"
|
|
|
|
#include <memory>
|
|
|
|
#include "base/environment.h"
|
|
#include "base/strings/string_util.h"
|
|
#include "base/threading/thread_restrictions.h"
|
|
#include "dbus/bus.h"
|
|
#include "dbus/message.h"
|
|
#include "dbus/object_proxy.h"
|
|
#include "ui/base/x/x11_util.h"
|
|
#include "ui/gfx/x/x11_atom_cache.h"
|
|
#include "ui/gfx/x/xproto.h"
|
|
#include "ui/gfx/x/xproto_util.h"
|
|
|
|
namespace electron {
|
|
|
|
void SetWMSpecState(x11::Window window, bool enabled, x11::Atom state) {
|
|
ui::SendClientMessage(window, ui::GetX11RootWindow(),
|
|
x11::GetAtom("_NET_WM_STATE"),
|
|
{enabled ? 1 : 0, static_cast<uint32_t>(state),
|
|
static_cast<uint32_t>(x11::Window::None), 1, 0});
|
|
}
|
|
|
|
void SetWindowType(x11::Window window, const std::string& type) {
|
|
std::string type_prefix = "_NET_WM_WINDOW_TYPE_";
|
|
x11::Atom window_type = x11::GetAtom(type_prefix + base::ToUpperASCII(type));
|
|
x11::SetProperty(window, x11::GetAtom("_NET_WM_WINDOW_TYPE"), x11::Atom::ATOM,
|
|
window_type);
|
|
}
|
|
|
|
bool ShouldUseGlobalMenuBar() {
|
|
base::ThreadRestrictions::ScopedAllowIO allow_io;
|
|
std::unique_ptr<base::Environment> env(base::Environment::Create());
|
|
if (env->HasVar("ELECTRON_FORCE_WINDOW_MENU_BAR"))
|
|
return false;
|
|
|
|
dbus::Bus::Options options;
|
|
scoped_refptr<dbus::Bus> bus(new dbus::Bus(options));
|
|
|
|
dbus::ObjectProxy* object_proxy =
|
|
bus->GetObjectProxy(DBUS_SERVICE_DBUS, dbus::ObjectPath(DBUS_PATH_DBUS));
|
|
dbus::MethodCall method_call(DBUS_INTERFACE_DBUS, "ListNames");
|
|
std::unique_ptr<dbus::Response> response(object_proxy->CallMethodAndBlock(
|
|
&method_call, dbus::ObjectProxy::TIMEOUT_USE_DEFAULT));
|
|
if (!response) {
|
|
bus->ShutdownAndBlock();
|
|
return false;
|
|
}
|
|
|
|
dbus::MessageReader reader(response.get());
|
|
dbus::MessageReader array_reader(nullptr);
|
|
if (!reader.PopArray(&array_reader)) {
|
|
bus->ShutdownAndBlock();
|
|
return false;
|
|
}
|
|
while (array_reader.HasMoreData()) {
|
|
std::string name;
|
|
if (array_reader.PopString(&name) &&
|
|
name == "com.canonical.AppMenu.Registrar") {
|
|
bus->ShutdownAndBlock();
|
|
return true;
|
|
}
|
|
}
|
|
|
|
bus->ShutdownAndBlock();
|
|
return false;
|
|
}
|
|
|
|
void MoveWindowToForeground(x11::Window window) {
|
|
MoveWindowAbove(window, static_cast<x11::Window>(0));
|
|
}
|
|
|
|
void MoveWindowAbove(x11::Window window, x11::Window other_window) {
|
|
ui::SendClientMessage(window, ui::GetX11RootWindow(),
|
|
x11::GetAtom("_NET_RESTACK_WINDOW"),
|
|
{2, static_cast<uint32_t>(other_window),
|
|
static_cast<uint32_t>(x11::StackMode::Above), 0, 0});
|
|
}
|
|
|
|
bool IsWindowValid(x11::Window window) {
|
|
auto* conn = x11::Connection::Get();
|
|
return conn->GetWindowAttributes({window}).Sync();
|
|
}
|
|
|
|
} // namespace electron
|