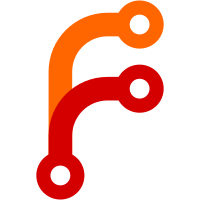
* [ci skip] refactor: create request context from network context * [ci skip] refactor: subscribe to mojo cookiemanager for cookie changes * [ci skip] refactor: manage the lifetime of custom URLRequestJobFactory * refactor: use OOP mojo proxy resolver * revert: add support for kIgnoreCertificateErrorsSPKIList * build: provide service manifest overlays for content services * chore: gn format * fix: log-net-log switch not working as expected * spec: verify proxy settings are respected from pac script with session.setProxy * chore: use chrome constants where possible * fix: initialize request context for global cert fetcher * refactor: fix destruction of request context getters * spec: use custom session for proxy tests * fix: queue up additional stop callbacks while net log is being stopped * fix: Add CHECK for cookie manager retrieval * chore: add helper to retrieve logging state for net log module * fix: ui::ResourceBundle::GetRawDataResourceForScale => GetRawDataResource * style: comment unused parameters * build: move //components/certificate_transparency deps from //brightray * chore: update gritsettings_resource_ids patch * chore: update api for chromium 68 * fix: net log instance is now a property of session
96 lines
3.2 KiB
C++
96 lines
3.2 KiB
C++
// Copyright (c) 2018 GitHub, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#include "atom/browser/net/resolve_proxy_helper.h"
|
|
|
|
#include "atom/browser/atom_browser_context.h"
|
|
#include "base/bind.h"
|
|
#include "base/threading/thread_task_runner_handle.h"
|
|
#include "net/url_request/url_request_context.h"
|
|
#include "net/url_request/url_request_context_getter.h"
|
|
|
|
namespace atom {
|
|
|
|
ResolveProxyHelper::ResolveProxyHelper(AtomBrowserContext* browser_context)
|
|
: context_getter_(browser_context->GetRequestContext()),
|
|
original_thread_(base::ThreadTaskRunnerHandle::Get()) {}
|
|
|
|
ResolveProxyHelper::~ResolveProxyHelper() {
|
|
// Clear all pending requests if the ProxyService is still alive.
|
|
pending_requests_.clear();
|
|
}
|
|
|
|
void ResolveProxyHelper::ResolveProxy(const GURL& url,
|
|
const ResolveProxyCallback& callback) {
|
|
// Enqueue the pending request.
|
|
pending_requests_.push_back(PendingRequest(url, callback));
|
|
|
|
// If nothing is in progress, start.
|
|
if (pending_requests_.size() == 1)
|
|
StartPendingRequest();
|
|
}
|
|
|
|
void ResolveProxyHelper::StartPendingRequest() {
|
|
auto& pending_request = pending_requests_.front();
|
|
context_getter_->GetNetworkTaskRunner()->PostTask(
|
|
FROM_HERE, base::BindOnce(&ResolveProxyHelper::StartPendingRequestInIO,
|
|
base::Unretained(this), pending_request.url));
|
|
}
|
|
|
|
void ResolveProxyHelper::StartPendingRequestInIO(const GURL& url) {
|
|
auto* proxy_service =
|
|
context_getter_->GetURLRequestContext()->proxy_resolution_service();
|
|
// Start the request.
|
|
int result = proxy_service->ResolveProxy(
|
|
url, std::string(), &proxy_info_,
|
|
base::Bind(&ResolveProxyHelper::OnProxyResolveComplete,
|
|
base::RetainedRef(this)),
|
|
nullptr, nullptr, net::NetLogWithSource());
|
|
// Completed synchronously.
|
|
if (result != net::ERR_IO_PENDING)
|
|
OnProxyResolveComplete(result);
|
|
}
|
|
|
|
void ResolveProxyHelper::OnProxyResolveComplete(int result) {
|
|
DCHECK(!pending_requests_.empty());
|
|
|
|
std::string proxy;
|
|
if (result == net::OK)
|
|
proxy = proxy_info_.ToPacString();
|
|
|
|
original_thread_->PostTask(
|
|
FROM_HERE, base::BindOnce(&ResolveProxyHelper::SendProxyResult,
|
|
base::RetainedRef(this), proxy));
|
|
}
|
|
|
|
void ResolveProxyHelper::SendProxyResult(const std::string& proxy) {
|
|
DCHECK(!pending_requests_.empty());
|
|
|
|
const auto& completed_request = pending_requests_.front();
|
|
if (!completed_request.callback.is_null())
|
|
completed_request.callback.Run(proxy);
|
|
|
|
// Clear the current (completed) request.
|
|
pending_requests_.pop_front();
|
|
|
|
// Start the next request.
|
|
if (!pending_requests_.empty())
|
|
StartPendingRequest();
|
|
}
|
|
|
|
ResolveProxyHelper::PendingRequest::PendingRequest(
|
|
const GURL& url,
|
|
const ResolveProxyCallback& callback)
|
|
: url(url), callback(callback) {}
|
|
|
|
ResolveProxyHelper::PendingRequest::PendingRequest(
|
|
ResolveProxyHelper::PendingRequest&& pending_request) = default;
|
|
|
|
ResolveProxyHelper::PendingRequest::~PendingRequest() noexcept = default;
|
|
|
|
ResolveProxyHelper::PendingRequest& ResolveProxyHelper::PendingRequest::
|
|
operator=(ResolveProxyHelper::PendingRequest&& pending_request) noexcept =
|
|
default;
|
|
|
|
} // namespace atom
|