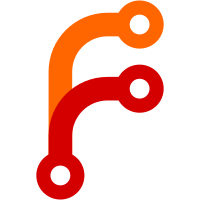
On OS X, the standard label that's used for the 'close' role is 'Close Window'. You can see this in the default macOS apps from Apple.
144 lines
3.1 KiB
JavaScript
144 lines
3.1 KiB
JavaScript
const {app} = require('electron')
|
|
|
|
const roles = {
|
|
about: {
|
|
get label () {
|
|
return process.platform === 'linux' ? 'About' : `About ${app.getName()}`
|
|
}
|
|
},
|
|
close: {
|
|
label: process.platform === 'darwin' ? 'Close Window' : 'Close',
|
|
accelerator: 'CommandOrControl+W',
|
|
windowMethod: 'close'
|
|
},
|
|
copy: {
|
|
label: 'Copy',
|
|
accelerator: 'CommandOrControl+C',
|
|
webContentsMethod: 'copy'
|
|
},
|
|
cut: {
|
|
label: 'Cut',
|
|
accelerator: 'CommandOrControl+X',
|
|
webContentsMethod: 'cut'
|
|
},
|
|
delete: {
|
|
label: 'Delete',
|
|
webContentsMethod: 'delete'
|
|
},
|
|
front: {
|
|
label: 'Bring All to Front'
|
|
},
|
|
help: {
|
|
label: 'Help'
|
|
},
|
|
hide: {
|
|
get label () {
|
|
return `Hide ${app.getName()}`
|
|
},
|
|
accelerator: 'Command+H'
|
|
},
|
|
hideothers: {
|
|
label: 'Hide Others',
|
|
accelerator: 'Command+Alt+H'
|
|
},
|
|
minimize: {
|
|
label: 'Minimize',
|
|
accelerator: 'CommandOrControl+M',
|
|
windowMethod: 'minimize'
|
|
},
|
|
paste: {
|
|
label: 'Paste',
|
|
accelerator: 'CommandOrControl+V',
|
|
webContentsMethod: 'paste'
|
|
},
|
|
pasteandmatchstyle: {
|
|
label: 'Paste and Match Style',
|
|
accelerator: 'Shift+CommandOrControl+V',
|
|
webContentsMethod: 'pasteAndMatchStyle'
|
|
},
|
|
quit: {
|
|
get label () {
|
|
switch (process.platform) {
|
|
case 'darwin': return `Quit ${app.getName()}`
|
|
case 'win32': return 'Exit'
|
|
default: return 'Quit'
|
|
}
|
|
},
|
|
accelerator: process.platform === 'win32' ? null : 'CommandOrControl+Q',
|
|
appMethod: 'quit'
|
|
},
|
|
redo: {
|
|
label: 'Redo',
|
|
accelerator: 'Shift+CommandOrControl+Z',
|
|
webContentsMethod: 'redo'
|
|
},
|
|
selectall: {
|
|
label: 'Select All',
|
|
accelerator: 'CommandOrControl+A',
|
|
webContentsMethod: 'selectAll'
|
|
},
|
|
services: {
|
|
label: 'Services'
|
|
},
|
|
togglefullscreen: {
|
|
label: 'Toggle Full Screen',
|
|
accelerator: process.platform === 'darwin' ? 'Control+Command+F' : 'F11',
|
|
windowMethod: function (window) {
|
|
window.setFullScreen(!window.isFullScreen())
|
|
}
|
|
},
|
|
undo: {
|
|
label: 'Undo',
|
|
accelerator: 'CommandOrControl+Z',
|
|
webContentsMethod: 'undo'
|
|
},
|
|
unhide: {
|
|
label: 'Show All'
|
|
},
|
|
window: {
|
|
label: 'Window'
|
|
},
|
|
zoom: {
|
|
label: 'Zoom'
|
|
}
|
|
}
|
|
|
|
exports.getDefaultLabel = (role) => {
|
|
if (roles.hasOwnProperty(role)) {
|
|
return roles[role].label
|
|
} else {
|
|
return ''
|
|
}
|
|
}
|
|
|
|
exports.getDefaultAccelerator = (role) => {
|
|
if (roles.hasOwnProperty(role)) return roles[role].accelerator
|
|
}
|
|
|
|
exports.execute = (role, focusedWindow, focusedWebContents) => {
|
|
if (!roles.hasOwnProperty(role)) return false
|
|
if (process.platform === 'darwin') return false
|
|
|
|
const {appMethod, webContentsMethod, windowMethod} = roles[role]
|
|
|
|
if (appMethod) {
|
|
app[appMethod]()
|
|
return true
|
|
}
|
|
|
|
if (windowMethod && focusedWindow != null) {
|
|
if (typeof windowMethod === 'function') {
|
|
windowMethod(focusedWindow)
|
|
} else {
|
|
focusedWindow[windowMethod]()
|
|
}
|
|
return true
|
|
}
|
|
|
|
if (webContentsMethod && focusedWebContents != null) {
|
|
focusedWebContents[webContentsMethod]()
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|