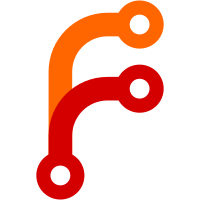
* Introduce `net.{start|stop}Logging()`
- Slight regression right now as Electron won't automatically start logging net-logs at launch, will soon be fixed
- To implement callback for async controls
* Add `net.isLogging` & optional callback param for `net.stopLogging()`
* Fix small regression on --log-net-log
--log-net-log should work again
* Error on empty file path
* Only start with valid file path
* Remove unused var
* Allow setting log file path before URLRequestContextGetter starts logging
* Add net log tests
* Remove redundant checks
* Use brightray::NetLog
* Clean up code
* Should automatically stop listening
* 🎨 Attempt to fix styles
* Only run non-null callback
* Dump file to tmpdir
* Simplify net log spec
Spawned Electron process on Linux CI can fail to launch
* Separate netLog module
* Remove net logging test from net spec
* Add tests for netLog
* Fix header guard
* Clean up code
* Add netLog.currentlyLoggingPath
* Callback with filepath
* Add test for case when only .stopLogging() is called
* Add docs
* Reintroduce error on invalid arg
* Update copyright
* Update error message
* Juggle file path string types
132 lines
3.9 KiB
C++
132 lines
3.9 KiB
C++
// Copyright (c) 2012 The Chromium Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style license that can be
|
|
// found in the LICENSE-CHROMIUM file.
|
|
|
|
#include "brightray/browser/browser_client.h"
|
|
|
|
#include "base/lazy_instance.h"
|
|
#include "base/path_service.h"
|
|
#include "brightray/browser/browser_context.h"
|
|
#include "brightray/browser/browser_main_parts.h"
|
|
#include "brightray/browser/devtools_manager_delegate.h"
|
|
#include "brightray/browser/media/media_capture_devices_dispatcher.h"
|
|
#include "brightray/browser/notification_presenter.h"
|
|
#include "brightray/browser/platform_notification_service.h"
|
|
#include "content/public/browser/browser_thread.h"
|
|
#include "content/public/common/url_constants.h"
|
|
|
|
using content::BrowserThread;
|
|
|
|
namespace brightray {
|
|
|
|
namespace {
|
|
|
|
BrowserClient* g_browser_client;
|
|
|
|
base::LazyInstance<std::string>::DestructorAtExit
|
|
g_io_thread_application_locale = LAZY_INSTANCE_INITIALIZER;
|
|
|
|
std::string g_application_locale;
|
|
|
|
void SetApplicationLocaleOnIOThread(const std::string& locale) {
|
|
DCHECK_CURRENTLY_ON(BrowserThread::IO);
|
|
g_io_thread_application_locale.Get() = locale;
|
|
}
|
|
|
|
} // namespace
|
|
|
|
// static
|
|
void BrowserClient::SetApplicationLocale(const std::string& locale) {
|
|
DCHECK_CURRENTLY_ON(BrowserThread::UI);
|
|
|
|
if (!BrowserThread::PostTask(
|
|
BrowserThread::IO, FROM_HERE,
|
|
base::BindOnce(&SetApplicationLocaleOnIOThread, locale))) {
|
|
g_io_thread_application_locale.Get() = locale;
|
|
}
|
|
g_application_locale = locale;
|
|
}
|
|
|
|
BrowserClient* BrowserClient::Get() {
|
|
return g_browser_client;
|
|
}
|
|
|
|
BrowserClient::BrowserClient() : browser_main_parts_(nullptr) {
|
|
DCHECK(!g_browser_client);
|
|
g_browser_client = this;
|
|
}
|
|
|
|
BrowserClient::~BrowserClient() {}
|
|
|
|
void BrowserClient::WebNotificationAllowed(
|
|
int render_process_id,
|
|
const base::Callback<void(bool, bool)>& callback) {
|
|
callback.Run(false, true);
|
|
}
|
|
|
|
NotificationPresenter* BrowserClient::GetNotificationPresenter() {
|
|
if (!notification_presenter_) {
|
|
// Create a new presenter if on OS X, Linux, or Windows 7+
|
|
notification_presenter_.reset(NotificationPresenter::Create());
|
|
}
|
|
return notification_presenter_.get();
|
|
}
|
|
|
|
BrowserMainParts* BrowserClient::OverrideCreateBrowserMainParts(
|
|
const content::MainFunctionParams&) {
|
|
return new BrowserMainParts;
|
|
}
|
|
|
|
content::BrowserMainParts* BrowserClient::CreateBrowserMainParts(
|
|
const content::MainFunctionParams& parameters) {
|
|
DCHECK(!browser_main_parts_);
|
|
browser_main_parts_ = OverrideCreateBrowserMainParts(parameters);
|
|
return browser_main_parts_;
|
|
}
|
|
|
|
content::MediaObserver* BrowserClient::GetMediaObserver() {
|
|
return MediaCaptureDevicesDispatcher::GetInstance();
|
|
}
|
|
|
|
content::PlatformNotificationService*
|
|
BrowserClient::GetPlatformNotificationService() {
|
|
if (!notification_service_)
|
|
notification_service_.reset(new PlatformNotificationService(this));
|
|
return notification_service_.get();
|
|
}
|
|
|
|
void BrowserClient::GetAdditionalAllowedSchemesForFileSystem(
|
|
std::vector<std::string>* additional_schemes) {
|
|
additional_schemes->push_back(content::kChromeDevToolsScheme);
|
|
additional_schemes->push_back(content::kChromeUIScheme);
|
|
}
|
|
|
|
void BrowserClient::GetAdditionalWebUISchemes(
|
|
std::vector<std::string>* additional_schemes) {
|
|
additional_schemes->push_back(content::kChromeDevToolsScheme);
|
|
}
|
|
|
|
NetLog* BrowserClient::GetNetLog() {
|
|
return &net_log_;
|
|
}
|
|
|
|
base::FilePath BrowserClient::GetDefaultDownloadDirectory() {
|
|
// ~/Downloads
|
|
base::FilePath path;
|
|
if (PathService::Get(base::DIR_HOME, &path))
|
|
path = path.Append(FILE_PATH_LITERAL("Downloads"));
|
|
|
|
return path;
|
|
}
|
|
|
|
content::DevToolsManagerDelegate* BrowserClient::GetDevToolsManagerDelegate() {
|
|
return new DevToolsManagerDelegate;
|
|
}
|
|
|
|
std::string BrowserClient::GetApplicationLocale() {
|
|
if (BrowserThread::CurrentlyOn(BrowserThread::IO))
|
|
return g_io_thread_application_locale.Get();
|
|
return g_application_locale;
|
|
}
|
|
|
|
} // namespace brightray
|