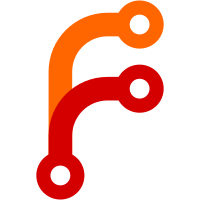
Closes #21666. This PR is fixing crashes caused by referencing and attempting to modify previously destroyed views. Before, when a view was destroyed and then the contents were referenced for modification, the system would crash as undefined memory was accessed. This fix explicitly makes the pointer to the destroyed view's contents null, so that this will not happen.
67 lines
1.8 KiB
C++
67 lines
1.8 KiB
C++
// Copyright (c) 2017 GitHub, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#ifndef SHELL_BROWSER_NATIVE_BROWSER_VIEW_H_
|
|
#define SHELL_BROWSER_NATIVE_BROWSER_VIEW_H_
|
|
|
|
#include <vector>
|
|
|
|
#include "base/macros.h"
|
|
#include "content/public/browser/web_contents.h"
|
|
#include "content/public/browser/web_contents_observer.h"
|
|
#include "third_party/skia/include/core/SkColor.h"
|
|
|
|
namespace gfx {
|
|
class Rect;
|
|
}
|
|
|
|
namespace electron {
|
|
|
|
enum AutoResizeFlags {
|
|
kAutoResizeWidth = 0x1,
|
|
kAutoResizeHeight = 0x2,
|
|
kAutoResizeHorizontal = 0x4,
|
|
kAutoResizeVertical = 0x8,
|
|
};
|
|
|
|
class InspectableWebContents;
|
|
class InspectableWebContentsView;
|
|
|
|
class NativeBrowserView : public content::WebContentsObserver {
|
|
public:
|
|
~NativeBrowserView() override;
|
|
|
|
static NativeBrowserView* Create(
|
|
InspectableWebContents* inspectable_web_contents);
|
|
|
|
InspectableWebContents* GetInspectableWebContents() {
|
|
return inspectable_web_contents_;
|
|
}
|
|
|
|
InspectableWebContentsView* GetInspectableWebContentsView();
|
|
content::WebContents* GetWebContents();
|
|
|
|
virtual void SetAutoResizeFlags(uint8_t flags) = 0;
|
|
virtual void SetBounds(const gfx::Rect& bounds) = 0;
|
|
virtual gfx::Rect GetBounds() = 0;
|
|
virtual void SetBackgroundColor(SkColor color) = 0;
|
|
|
|
// Called when the window needs to update its draggable region.
|
|
virtual void UpdateDraggableRegions(
|
|
const std::vector<gfx::Rect>& system_drag_exclude_areas) {}
|
|
|
|
protected:
|
|
explicit NativeBrowserView(InspectableWebContents* inspectable_web_contents);
|
|
// content::WebContentsObserver:
|
|
void WebContentsDestroyed() override;
|
|
|
|
InspectableWebContents* inspectable_web_contents_;
|
|
|
|
private:
|
|
DISALLOW_COPY_AND_ASSIGN(NativeBrowserView);
|
|
};
|
|
|
|
} // namespace electron
|
|
|
|
#endif // SHELL_BROWSER_NATIVE_BROWSER_VIEW_H_
|