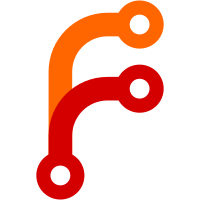
* chore: update to Node.js v18 * child_process: improve argument validation https://github.com/nodejs/node/pull/41305 * bootstrap: support configure-time user-land snapshot https://github.com/nodejs/node/pull/42466 * chore: update GN patch * src: disambiguate terms used to refer to builtins and addons https://github.com/nodejs/node/pull/44135 * src: use a typed array internally for process._exiting https://github.com/nodejs/node/pull/43883 * chore: lib/internal/bootstrap -> lib/internal/process * src: disambiguate terms used to refer to builtins and addons https://github.com/nodejs/node/pull/44135 * chore: remove redudant browserGlobals patch * chore: update BoringSSL patch * src: allow embedder-provided PageAllocator in NodePlatform https://github.com/nodejs/node/pull/38362 * chore: fixup Node.js crypto tests - https://github.com/nodejs/node/pull/44171 - https://github.com/nodejs/node/pull/41600 * lib: add Promise methods to avoid-prototype-pollution lint rule https://github.com/nodejs/node/pull/43849 * deps: update V8 to 10.1 https://github.com/nodejs/node/pull/42657 * src: add kNoBrowserGlobals flag for Environment https://github.com/nodejs/node/pull/40532 * chore: consolidate asar initialization patches * deps: update V8 to 10.1 https://github.com/nodejs/node/pull/42657 * deps: update V8 to 9.8 https://github.com/nodejs/node/pull/41610 * src,crypto: remove AllocatedBuffers from crypto_spkac https://github.com/nodejs/node/pull/40752 * build: enable V8's shared read-only heap https://github.com/nodejs/node/pull/42809 * src: fix ssize_t error from nghttp2.h https://github.com/nodejs/node/pull/44393 * chore: fixup ESM patch * chore: fixup patch indices * src: merge NativeModuleEnv into NativeModuleLoader https://github.com/nodejs/node/pull/43824 * [API] Pass OOMDetails to OOMErrorCallback https://chromium-review.googlesource.com/c/v8/v8/+/3647827 * src: iwyu in cleanup_queue.cc * src: return Maybe from a couple of functions https://github.com/nodejs/node/pull/39603 * src: clean up embedder API https://github.com/nodejs/node/pull/35897 * src: refactor DH groups to delete crypto_groups.h https://github.com/nodejs/node/pull/43896 * deps,src: use SIMD for normal base64 encoding https://github.com/nodejs/node/pull/39775 * chore: remove deleted source file * chore: update patches * chore: remove deleted source file * lib: add fetch https://github.com/nodejs/node/pull/41749 * chore: remove nonexistent node specs * test: split report OOM tests https://github.com/nodejs/node/pull/44389 * src: trace fs async api https://github.com/nodejs/node/pull/44057 * http: trace http request / response https://github.com/nodejs/node/pull/44102 * test: split test-crypto-dh.js https://github.com/nodejs/node/pull/40451 * crypto: introduce X509Certificate API https://github.com/nodejs/node/pull/36804 * src: split property helpers from node::Environment https://github.com/nodejs/node/pull/44056 * https://github.com/nodejs/node/pull/38905 bootstrap: implement run-time user-land snapshots via --build-snapshot and --snapshot-blob * lib,src: implement WebAssembly Web API https://github.com/nodejs/node/pull/42701 * fixup! deps,src: use SIMD for normal base64 encoding * fixup! src: refactor DH groups to delete crypto_groups.h * chore: fixup base64 GN file * fix: check that node::InitializeContext() returns true * chore: delete _noBrowserGlobals usage * chore: disable fetch in renderer procceses * dns: default to verbatim=true in dns.lookup() https://github.com/nodejs/node/pull/39987 Co-authored-by: PatchUp <73610968+patchup[bot]@users.noreply.github.com>
37 lines
1.3 KiB
C++
37 lines
1.3 KiB
C++
// Copyright (c) 2019 GitHub, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#include "shell/common/node_util.h"
|
|
|
|
#include "base/logging.h"
|
|
#include "shell/common/node_includes.h"
|
|
|
|
namespace electron::util {
|
|
|
|
v8::MaybeLocal<v8::Value> CompileAndCall(
|
|
v8::Local<v8::Context> context,
|
|
const char* id,
|
|
std::vector<v8::Local<v8::String>>* parameters,
|
|
std::vector<v8::Local<v8::Value>>* arguments,
|
|
node::Environment* optional_env) {
|
|
v8::Isolate* isolate = context->GetIsolate();
|
|
v8::TryCatch try_catch(isolate);
|
|
v8::MaybeLocal<v8::Function> compiled =
|
|
node::builtins::BuiltinLoader::LookupAndCompile(context, id, parameters,
|
|
optional_env);
|
|
if (compiled.IsEmpty()) {
|
|
return v8::MaybeLocal<v8::Value>();
|
|
}
|
|
v8::Local<v8::Function> fn = compiled.ToLocalChecked().As<v8::Function>();
|
|
v8::MaybeLocal<v8::Value> ret = fn->Call(
|
|
context, v8::Null(isolate), arguments->size(), arguments->data());
|
|
// This will only be caught when something has gone terrible wrong as all
|
|
// electron scripts are wrapped in a try {} catch {} by webpack
|
|
if (try_catch.HasCaught()) {
|
|
LOG(ERROR) << "Failed to CompileAndCall electron script: " << id;
|
|
}
|
|
return ret;
|
|
}
|
|
|
|
} // namespace electron::util
|