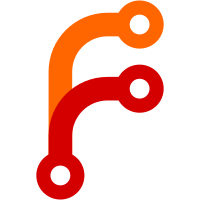
* rebase "feat: enable windows control overlay on Windows" * correct compilation error * fix linting errors * modify includes and build file * change `hidden` option to `overlay` * add patch to fix visual layout * add button background color parameter * add button text color parameter * modify `overlay` in docs and modify button hover/press transition color * change `text` to `symbol` * remove todo and fix `text` replacement * add new titleBarOverlay property and remove titleBarStyle `overlay` * update browser and frameless window docs * remove chromium patches * chore: update patches * change button hover color, update trailing `_`, update test file * add dchecks, update title bar drawing checks, update test file * modify for mac and linux builds * update docs with overlayColor and overlaySymbolColor * add corner and side hit test info * modify docs and copyright info * modify `titlebar_overlay_` as boolean or object * move `title_bar_style_ to `NativeWindow` * update docs with boolean and object titlebar_overlay_ * add `IsEmpty` checks * move get options for boolean and object checks * fix linting error * disable `use_lld` for macos * Update docs/api/frameless-window.md Co-authored-by: John Kleinschmidt <jkleinsc@electronjs.org> * Update docs/api/frameless-window.md Co-authored-by: John Kleinschmidt <jkleinsc@electronjs.org> * Update docs/api/frameless-window.md Co-authored-by: John Kleinschmidt <jkleinsc@electronjs.org> * Apply docs suggestions from code review Co-authored-by: Jeremy Rose <jeremya@chromium.org> * modify `true` option description `titleBarOverlay` * ci: cleanup keychain after tests on arm64 mac (#30472) Co-authored-by: John Kleinschmidt <jkleinsc@electronjs.org> Co-authored-by: PatchUp <73610968+patchup[bot]@users.noreply.github.com> Co-authored-by: Jeremy Rose <jeremya@chromium.org>
59 lines
1.6 KiB
C++
59 lines
1.6 KiB
C++
// Copyright (c) 2014 GitHub, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#ifndef SHELL_BROWSER_UI_VIEWS_FRAMELESS_VIEW_H_
|
|
#define SHELL_BROWSER_UI_VIEWS_FRAMELESS_VIEW_H_
|
|
|
|
#include "ui/views/window/non_client_view.h"
|
|
|
|
namespace views {
|
|
class Widget;
|
|
}
|
|
|
|
namespace electron {
|
|
|
|
class NativeWindowViews;
|
|
|
|
class FramelessView : public views::NonClientFrameView {
|
|
public:
|
|
static const char kViewClassName[];
|
|
FramelessView();
|
|
~FramelessView() override;
|
|
|
|
virtual void Init(NativeWindowViews* window, views::Widget* frame);
|
|
|
|
// Returns whether the |point| is on frameless window's resizing border.
|
|
int ResizingBorderHitTest(const gfx::Point& point);
|
|
|
|
protected:
|
|
// views::NonClientFrameView:
|
|
gfx::Rect GetBoundsForClientView() const override;
|
|
gfx::Rect GetWindowBoundsForClientBounds(
|
|
const gfx::Rect& client_bounds) const override;
|
|
int NonClientHitTest(const gfx::Point& point) override;
|
|
void GetWindowMask(const gfx::Size& size, SkPath* window_mask) override;
|
|
void ResetWindowControls() override;
|
|
void UpdateWindowIcon() override;
|
|
void UpdateWindowTitle() override;
|
|
void SizeConstraintsChanged() override;
|
|
|
|
// Overridden from View:
|
|
gfx::Size CalculatePreferredSize() const override;
|
|
gfx::Size GetMinimumSize() const override;
|
|
gfx::Size GetMaximumSize() const override;
|
|
const char* GetClassName() const override;
|
|
|
|
// Not owned.
|
|
NativeWindowViews* window_ = nullptr;
|
|
views::Widget* frame_ = nullptr;
|
|
|
|
friend class NativeWindowsViews;
|
|
|
|
private:
|
|
DISALLOW_COPY_AND_ASSIGN(FramelessView);
|
|
};
|
|
|
|
} // namespace electron
|
|
|
|
#endif // SHELL_BROWSER_UI_VIEWS_FRAMELESS_VIEW_H_
|