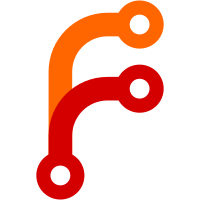
* [ci skip] refactor: create request context from network context * [ci skip] refactor: subscribe to mojo cookiemanager for cookie changes * [ci skip] refactor: manage the lifetime of custom URLRequestJobFactory * refactor: use OOP mojo proxy resolver * revert: add support for kIgnoreCertificateErrorsSPKIList * build: provide service manifest overlays for content services * chore: gn format * fix: log-net-log switch not working as expected * spec: verify proxy settings are respected from pac script with session.setProxy * chore: use chrome constants where possible * fix: initialize request context for global cert fetcher * refactor: fix destruction of request context getters * spec: use custom session for proxy tests * fix: queue up additional stop callbacks while net log is being stopped * fix: Add CHECK for cookie manager retrieval * chore: add helper to retrieve logging state for net log module * fix: ui::ResourceBundle::GetRawDataResourceForScale => GetRawDataResource * style: comment unused parameters * build: move //components/certificate_transparency deps from //brightray * chore: update gritsettings_resource_ids patch * chore: update api for chromium 68 * fix: net log instance is now a property of session
46 lines
1.4 KiB
C++
46 lines
1.4 KiB
C++
// Copyright (c) 2015 GitHub, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#include "atom/utility/atom_content_utility_client.h"
|
|
|
|
#include "content/public/child/child_thread.h"
|
|
#include "services/proxy_resolver/proxy_resolver_service.h"
|
|
#include "services/proxy_resolver/public/mojom/proxy_resolver.mojom.h"
|
|
|
|
#if defined(OS_WIN)
|
|
#include "chrome/utility/printing_handler_win.h"
|
|
#endif
|
|
|
|
namespace atom {
|
|
|
|
AtomContentUtilityClient::AtomContentUtilityClient() {
|
|
#if defined(OS_WIN)
|
|
handlers_.push_back(std::make_unique<printing::PrintingHandlerWin>());
|
|
#endif
|
|
}
|
|
|
|
AtomContentUtilityClient::~AtomContentUtilityClient() {}
|
|
|
|
bool AtomContentUtilityClient::OnMessageReceived(const IPC::Message& message) {
|
|
#if defined(OS_WIN)
|
|
for (const auto& handler : handlers_) {
|
|
if (handler->OnMessageReceived(message))
|
|
return true;
|
|
}
|
|
#endif
|
|
|
|
return false;
|
|
}
|
|
|
|
void AtomContentUtilityClient::RegisterServices(StaticServiceMap* services) {
|
|
service_manager::EmbeddedServiceInfo proxy_resolver_info;
|
|
proxy_resolver_info.task_runner =
|
|
content::ChildThread::Get()->GetIOTaskRunner();
|
|
proxy_resolver_info.factory =
|
|
base::BindRepeating(&proxy_resolver::ProxyResolverService::CreateService);
|
|
services->emplace(proxy_resolver::mojom::kProxyResolverServiceName,
|
|
proxy_resolver_info);
|
|
}
|
|
|
|
} // namespace atom
|